controller返回file下载
时间: 2024-04-10 14:34:04 浏览: 28
好的,下面是一个示例代码,展示了如何在一个Spring Boot应用程序中使用Controller返回文件并进行下载:
```java
import org.springframework.core.io.Resource;
import org.springframework.core.io.UrlResource;
import org.springframework.http.HttpHeaders;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RestController;
import java.io.IOException;
import java.net.MalformedURLException;
import java.nio.file.Path;
import java.nio.file.Paths;
@RestController
public class FileController {
private static final String FILE_DIRECTORY = "path/to/your/file/directory/";
@GetMapping("/download/{filename}")
public ResponseEntity<Resource> downloadFile(@PathVariable String filename) {
// 构建文件路径
Path filePath = Paths.get(FILE_DIRECTORY).resolve(filename).normalize();
try {
// 加载文件资源
Resource resource = new UrlResource(filePath.toUri());
if (resource.exists()) {
// 设置响应头
HttpHeaders headers = new HttpHeaders();
headers.add(HttpHeaders.CONTENT_DISPOSITION, "attachment; filename=" + resource.getFilename());
// 返回文件响应
return ResponseEntity.ok()
.headers(headers)
.contentType(MediaType.APPLICATION_OCTET_STREAM)
.body(resource);
}
} catch (MalformedURLException e) {
e.printStackTrace();
}
// 如果文件不存在,则返回404错误
return ResponseEntity.notFound().build();
}
}
```
在上述示例中,您需要将`path/to/your/file/directory/`替换为您实际文件存储的路径。当客户端发起`/download/{filename}`的GET请求时,该方法将根据文件名构建文件路径,并使用`UrlResource`加载文件资源。如果文件存在,将设置适当的响应头,并返回文件内容作为响应体。如果文件不存在,则返回404错误。
请注意,上述示例使用的是Spring Boot框架,但您可以根据自己的需求进行适当的修改,以在其他框架或平台上实现相似的功能。
希望对您有所帮助!如果您还有其他问题,请随时提问。
相关推荐
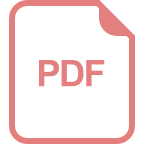
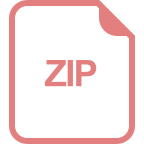
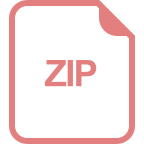














