离合器多目标优化算法代码
时间: 2024-08-14 13:04:45 浏览: 101
离合器多目标优化算法是一种用于解决多目标优化问题的搜索策略,它通常结合了遗传算法(Genetic Algorithm, GA)与离散离合器模型。离合器原理模拟了车辆换挡过程中的离合器控制,通过切换不同的解决方案来寻找最佳平衡点。
在编写代码时,你可以按照以下步骤:
1. **导入库**:
首先,导入必要的数学库(如numpy、scipy)、优化库(如deap)以及离合器模型相关的模块。
```python
import numpy as np
from deap import base, creator, tools
```
2. **设定问题定义**:
定义问题的目标函数和约束条件,以及离合器的工作模式(例如,单目标离合器或多阶段离合器)。
```python
creator.create("FitnessMulti", base.Fitness, weights=(-1.0, -1.0)) # 可能需要调整权重
creator.create("Individual", list, fitness=creator.FitnessMulti)
def objectives(individual):
# 这里是你定义的目标函数
...
```
3. **初始化种群**:
创建一个种群并随机生成初始个体。
```python
toolbox = base.Toolbox()
toolbox.register("attr_float", random.uniform, -10, 10)
toolbox.register("individual", tools.initRepeat, creator.Individual, toolbox.attr_float, n=len(individual_size))
```
4. **离合器操作**:
实现适应度评估和选择过程中离合器的工作机制,比如轮盘赌选择和精英保留等。
```python
def clutch_selection(population, offspring, current_best):
# 调整部分个体以模拟离合器切换
...
```
5. **算法循环**:
主循环包含进化算子(选择、交叉、变异)以及离合器操作。
```python
while not stopping_condition:
offspring = toolbox.select(population, len(offspring))
offspring = clutch_selection(population, offspring, current_best)
offspring = toolbox.mutate(offspring) # 变异操作
# 进行交叉和其他操作...
population[:] = toolbox.evolve(population=offspring)
```
6. **结果处理**:
从最终种群中提取并展示非支配解集。
```python
non_dominated_solutions = tools.sortNondominated(population, k=len(population), first_front_only=True)
```
阅读全文
相关推荐
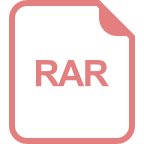
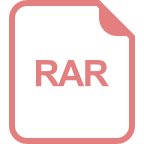
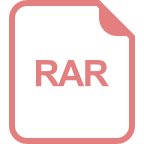















