C语言中二、查找并输出一个字符串中最长的单词。不同的单词之间以非字母字符分隔。正确程序的输出结果如下:字符串I am a student. I come from China! 中第一个最长单词为: student。
时间: 2024-12-23 09:16:08 浏览: 12
在C语言中,寻找并输出字符串中最长的单词需要一些步骤。首先,你需要遍历整个字符串,然后分割字符串成单个单词。这里我们可以假设非字母字符如空格、标点符号等作为分隔符。接下来是一个简单的流程:
1. 定义一个变量来存储当前找到的最长单词和它的长度。
2. 初始化这两个值为第一个字符及其长度(因为如果字符串的第一个字符就是一个完整的单词,它将是初始最长单词)。
3. 遍历字符串,对于每个字符,检查是否是非字母字符:
- 如果是,检查当前位置和上一个字母字符之间的子串是否比已知的最长单词还要长。如果是,则更新最长单词和其长度。
- 如果不是,将当前字符加入到当前单词中,并继续检查下一个字符。
4. 当遍历完整个字符串后,最长单词就会储存在初始定义的变量中。
下面是一个简化的C代码示例来实现这个功能:
```c
#include <stdio.h>
#include <ctype.h>
#include <string.h>
#define MAX_WORD_LENGTH 100
void find_longest_word(char *str) {
char current_word[MAX_WORD_LENGTH] = "";
int word_length = 0;
int longest_word_length = 0;
char last_char = '\0'; // 初始化最后一个字符为'\0'
for (int i = 0; str[i]; ++i) { // 遍历字符串
if (!isalpha(str[i])) { // 检查非字母字符
if (word_length > longest_word_length) {
strncpy(current_word, last_char, word_length);
current_word[word_length] = '\0';
longest_word_length = word_length;
}
word_length = 0; // 清空当前单词
last_char = '\0'; // 重置上一个字符
} else { // 如果是字母字符
current_word[word_length++] = str[i];
last_char = str[i];
}
}
// 检查最后一个单词
if (word_length > longest_word_length) {
strncpy(current_word, last_char, word_length);
current_word[word_length] = '\0';
longest_word_length = word_length;
}
printf("最长的单词为: %s\n", current_word); // 输出结果
}
int main() {
const char *str = "I am a student. I come from China!";
find_longest_word(str);
return 0;
}
```
当你运行此程序,它会输出字符串中最长的单词"student"。
阅读全文
相关推荐
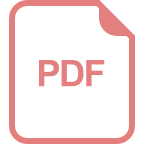
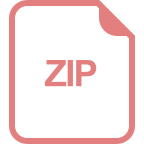
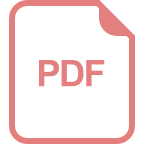

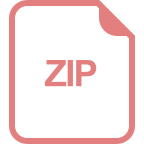














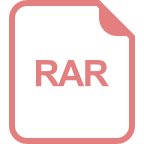