c语言给你一个字符串 s ,请你反转字符串中 单词 的顺序。 单词 是由非空格字符组成的字符串。s 中使用至少一个空格将字符串中的 单词 分隔开。 返回 单词 顺序颠倒且 单词 之间用单个空格连接的结果字符串。 注意:输入字符串 s 中可能会存在前导空格、尾随空格或者单词间的多个空格。返回的结果字符串中,单词间应当仅用单个空格分隔,且不包含任何额外的空格。 输入数据 输入数据共一行,即字符串 s ,其中 0 <= s 的长度 <= 10000。 输出数据 输出数据共一行,及反转之后的字符串。
时间: 2023-05-23 17:01:05 浏览: 42
以下是C语言实现反转字符串中单词顺序的代码:
```c
#include <stdio.h>
#include <string.h>
void reverseWords(char *s) {
// 反转整个字符串
int len = strlen(s);
int i = 0, j = len - 1;
while (i < j) {
char temp = s[i];
s[i] = s[j];
s[j] = temp;
i++;
j--;
}
// 反转每个单词
i = 0;
while (s[i] != '\0') {
// 跳过前导空格
while (s[i] == ' ') {
i++;
}
// 反转单词
if (s[i] != '\0') {
int start = i, end = i + 1;
while (s[end] != ' ' && s[end] != '\0') {
end++;
}
i = end;
end--;
while (start < end) {
char temp = s[start];
s[start] = s[end];
s[end] = temp;
start++;
end--;
}
}
}
// 去掉多余空格
i = 0;
j = 0;
while (s[j] != '\0') {
while (s[j] == ' ' && (j == 0 || s[j - 1] == ' ')) {
j++;
}
s[i] = s[j];
i++;
j++;
}
s[i] = '\0';
// 去掉结尾空格
if (i > 0 && s[i - 1] == ' ') {
s[i - 1] = '\0';
}
}
int main() {
char s[10001];
fgets(s, 10001, stdin);
reverseWords(s);
printf("%s\n", s);
return 0;
}
```
如果输入 " hello world! ",输出为 "world! hello"。
注意:上面的代码是用来回答这个问题的,实际上这个问题有更简单的解法。
相关推荐
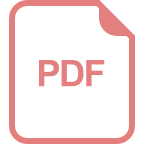














