python 收发文本文件
时间: 2023-10-13 18:18:47 浏览: 228
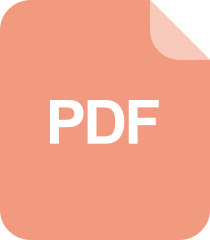
python创建文本文件的简单方法
要在Python中收发文本文件,你可以使用文件处理和网络通信的相关模块。以下是一些基本的步骤:
1. 发送方:
- 打开要发送的文本文件,并读取其内容。
- 使用套接字(socket)模块建立网络连接。
- 使用套接字的发送方法,将文本数据发送给接收方。
2. 接收方:
- 使用套接字模块建立网络连接。
- 使用套接字的接收方法,接收来自发送方的数据。
- 打开要保存接收到的文本文件,并将接收到的数据写入文件。
这是一个简单的例子,演示了如何使用Python中的socket模块实现文本文件的发送和接收:
发送方代码(sender.py):
```python
import socket
def send_file(filename, host, port):
with open(filename, 'r') as file:
data = file.read()
with socket.socket() as s:
s.connect((host, port))
s.sendall(data.encode())
filename = 'test.txt'
host = '127.0.0.1'
port = 12345
send_file(filename, host, port)
```
接收方代码(receiver.py):
```python
import socket
def receive_file(filename, host, port):
with socket.socket() as s:
s.bind((host, port))
s.listen(1)
conn, addr = s.accept()
with open(filename, 'w') as file:
while True:
data = conn.recv(1024)
if not data:
break
file.write(data.decode())
filename = 'received.txt'
host = '127.0.0.1'
port = 12345
receive_file(filename, host, port)
```
上述代码中,发送方将文件test.txt的内容发送到接收方的主机和端口。接收方会接收数据,并将其写入received.txt文件中。
请确保接收方在发送方发送文件之前启动,以便能够接收到数据。还要注意,这只是一个简单的示例,没有进行错误处理或完整性检查,请根据实际情况进行改进。
阅读全文
相关推荐
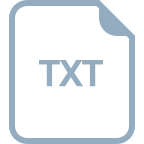
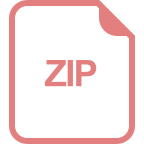
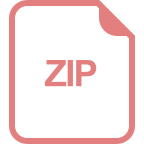
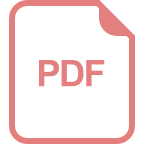
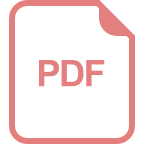
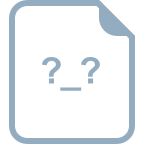
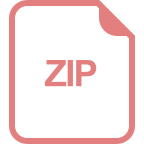
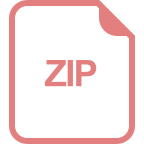
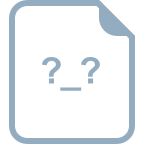
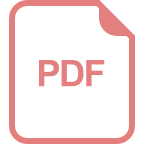
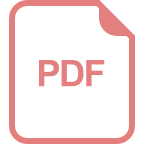
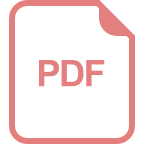
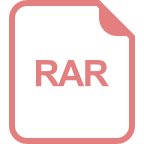
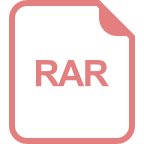
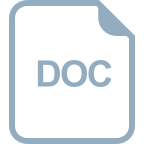
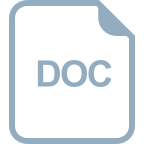
