springcloud整合grpc
时间: 2025-01-04 08:12:02 浏览: 5
### Spring Cloud 中集成 gRPC 服务
#### Maven 配置
为了在 Spring Cloud 项目中成功集成 gRPC,首先需要设置项目的 `pom.xml` 文件来引入必要的依赖项。这通常涉及到添加特定于 gRPC 和 Protocol Buffers 编译器插件的支持[^4]。
```xml
<dependencies>
<!-- gRPC dependency -->
<dependency>
<groupId>io.grpc</groupId>
<artifactId>grpc-netty-shaded</artifactId>
<version>${grpc.version}</version>
</dependency>
<!-- Protobuf Java runtime library and compiler plugin -->
<dependency>
<groupId>com.google.protobuf</groupId>
<artifactId>protobuf-java</artifactId>
<version>${protobuf.version}</version>
</dependency>
<!-- Other dependencies... -->
</dependencies>
<!-- Plugin configuration for compiling .proto files into Java classes -->
<build>
<extensions>
<extension>
<groupId>kr.motd.maven</groupId>
<artifactId>os-maven-plugin</artifactId>
<version>1.6.2</version>
</extension>
</extensions>
<plugins>
<plugin>
<groupId>org.xolstice.maven.plugins</groupId>
<artifactId>protobuf-maven-plugin</artifactId>
<version>0.6.1</version>
<configuration>
<protocArtifact>com.google.protobuf:protoc:${protobuf.version}:exe:${os.detected.classifier}</protocArtifact>
<pluginId>grpc-java</pluginId>
<pluginArtifact>io.grpc:protoc-gen-grpc-java:${grpc.version}:exe:${os.detected.classifier}</pluginArtifact>
</configuration>
<executions>
<execution>
<goals>
<goal>compile</goal>
<goal>compile-custom</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
```
#### 定义 Proto 文件
创建 `.proto` 文件用于描述服务接口以及消息结构。这些文件应当放置在一个名为 `common` 的模块内以便被其他模块所共享使用。
```protobuf
syntax = "proto3";
option java_multiple_files = true;
package com.example;
service ExampleService {
rpc SayHello (HelloRequest) returns (HelloResponse);
}
message HelloRequest {
string name = 1;
}
message HelloResponse {
string message = 1;
}
```
#### YAML 配置
对于服务器端而言,在应用程序的配置文件 (`application.yml`) 中指定监听地址和其他必要参数;而对于客户端,则需指明目标主机名和端口,并可选地设定连接选项如协商类型为纯文本模式以简化开发过程。
**Server-side Configuration**
```yaml
server:
port: 9090
grpc:
server:
port: ${server.port}
```
**Client-side Configuration**
```yaml
spring:
application:
name: example-client
grpc:
negotiation-type: plaintext
name-resolver-provider: dns-unresolved
enable-channelz: false
keepalive-time: 1h
max-inbound-message-size: 4MB
```
#### 实现 Service 类
编写具体的业务逻辑处理程序并将其注册到 gRPC 服务器上作为远程调用的目标对象实例之一。
```java
@Service
public class ExampleServiceImpl extends ExampleGrpc.ExampleImplBase {
@Override
public void sayHello(HelloRequest request, StreamObserver<HelloResponse> responseObserver) {
String greeting = new StringBuilder().append("Hello ").append(request.getName()).toString();
HelloResponse reply = HelloResponse.newBuilder()
.setMessage(greeting).build();
responseObserver.onNext(reply);
responseObserver.onCompleted();
}
}
```
#### 启动 Server 和 Client 应用
最后一步是在各自的应用入口处启动相应的 gRPC 组件——通过继承自 `SpringBootServletInitializer` 或者直接利用 `@SpringBootApplication` 注解标记主类的方式完成初始化工作。
```java
@SpringBootApplication
@EnableDiscoveryClient
public class GrpcApplication {
private static final Logger logger = LoggerFactory.getLogger(GrpcApplication.class);
public static void main(String[] args) throws Exception {
SpringApplication.run(GrpcApplication.class, args);
}
@Bean
public ServerFactoryBean createGrpcServer() {
return NettyServerBuilder.forPort(port)
.addService(new ExampleServiceImpl())
.build();
}
}
```
阅读全文
相关推荐















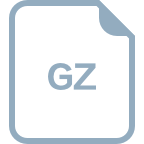


