python避免死锁
时间: 2024-08-27 17:03:40 浏览: 87
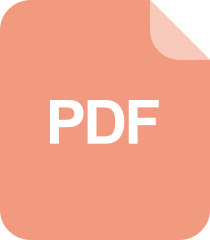
python避免死锁方法实例分析
在Python中,由于其GIL全局解释器锁的存在,通常不会遇到传统意义上的死锁问题,因为同一时间只有一个线程在执行Python字节码。然而,尽管如此,仍然有其他情况可能导致性能瓶颈,而不是死锁:
1. 竞争条件(Race Condition):如果两个或多个线程对共享资源进行无序访问,可能会导致数据竞争,但并不会形成典型的“等待资源然后请求已被其他线程持有的资源”的死锁模式。
2. 长期占用资源:虽然不是死锁,但长时间持有锁而不释放可能导致资源浪费和性能下降,尤其是在高并发场景下。
为了避免这类问题,Python开发者可以采取以下策略:
- 尽量减少全局变量和同步操作,特别是对于需要多个线程共享的数据。
- 使用`with`语句处理文件、数据库连接等资源,确保它们在离开作用域时自动关闭。
- 对于长时间运行的任务,考虑使用异步IO或者进程池来提高并发效率。
- 使用适当的锁策略,比如读写锁(Reader-Writer Locks),在不需要互斥访问时允许更多的读取者进入。
阅读全文
相关推荐
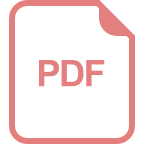
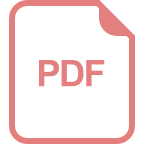
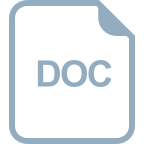
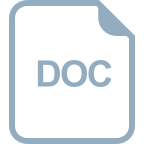
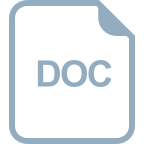
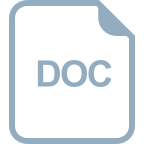
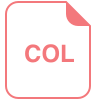
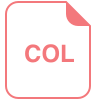
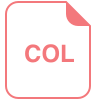
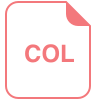
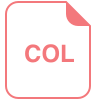
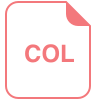
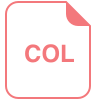



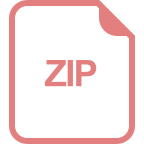