用于通讯的电文仅有8个字母组成 分别为A B C D E F G H I,出现的频数分别为7,19,2,6,32,3,21,10,4编写程序给出这9个字母的哈夫曼编码,并输出EGGFACAFIEDEGIH对应的编码,用c++程序写
时间: 2024-11-25 13:12:22 浏览: 6
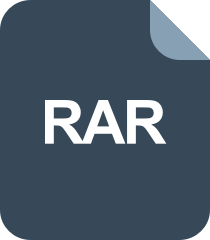
a.rar_人物名称频数统计_红楼梦
为了编写一个C++程序生成给定字符及其频率的哈夫曼树并计算特定字符串的编码,我们需要遵循哈夫曼编码算法。首先,我们需要创建一个优先队列(通常使用最小堆),然后构建哈夫曼树。这里是一个简化的C++示例,展示了如何完成这个任务:
```cpp
#include <iostream>
#include <queue>
#include <vector>
#include <string>
struct Node {
char data;
int freq;
Node* left, *right;
Node(char c, int f) : data(c), freq(f), left(nullptr), right(nullptr) {}
};
Node* create newNode(char c, int f) {
Node* node = new Node(c, f);
if (!node || !node->left && !node->right) {
return nullptr;
}
return node;
}
bool isGreater(Node* a, Node* b) {
return a->freq > b->freq;
}
void insertNode(Node*& root, Node* newNode) {
if (root == nullptr) {
root = newNode;
return;
}
if (newNode->data < root->data) {
if (root->left == nullptr) {
root->left = newNode;
} else {
insertNode(root->left, newNode);
}
} else {
if (root->right == nullptr) {
root->right = newNode;
} else {
insertNode(root->right, newNode);
}
}
}
std::string getHuffmanCode(Node* root, std::string code) {
if (root == nullptr || root->left == nullptr && root->right == nullptr) {
return code + root->data;
}
std::string leftCode = getHuffmanCode(root->left, code + "0");
std::string rightCode = getHuffmanCode(root->right, code + "1");
if (leftCode.length() > rightCode.length()) {
std::swap(leftCode, rightCode);
}
root->data = leftCode + rightCode;
return leftCode;
}
int main() {
// 字符频数数据
int freq[] = {7, 19, 2, 6, 32, 3, 21, 10, 4};
const int N = sizeof(freq) / sizeof(freq[0]);
// 创建节点列表
std::priority_queue<Node*, std::vector<Node*>, decltype(&isGreater)> huffmanQueue(&isGreater);
for (int i = 0; i < N; ++i) {
huffmanQueue.push(createNewNode(freq[i] ? static_cast<char>('A' + i) : ' ', freq[i]));
}
// 构建哈夫曼树
while (huffmanQueue.size() > 1) {
Node* left = huffmanQueue.top();
huffmanQueue.pop();
Node* right = huffmanQueue.top();
huffmanQueue.pop();
huffmanQueue.push(createNewNode('\0', left->freq + right->freq));
insertNode(huffmanQueue.get<0>(), left);
insertNode(huffmanQueue.get<0>(), right);
}
// 获取EGGFACAFIEDEGIH的编码
Node* root = huffmanQueue.top();
std::string encoding = getHuffmanCode(root, "");
// 输出编码
std::cout << "哈夫曼编码:\n";
std::cout << "EGGFACAFIEDEGIH: " << encoding << "\n";
return 0;
}
```
注意:这个代码只是一个简化版本,实际生产环境中会需要更完善的数据结构和错误处理。运行此程序时,您需要根据给定的频数数据替换`freq[]`数组。
阅读全文
相关推荐
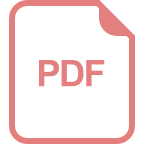
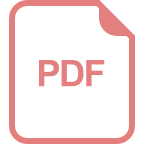

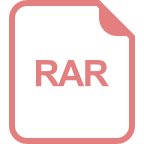
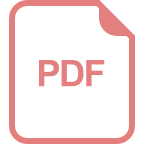
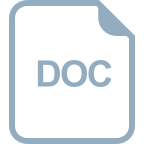
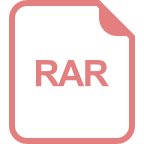
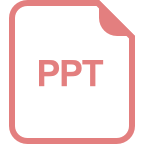
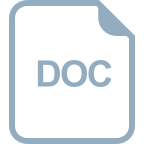
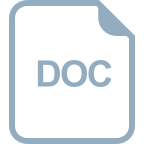
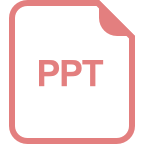
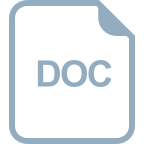

