用python中的words函数编写一个程序,查找用户输入字符串中每个字母的出现位置,并输入结果
时间: 2024-10-22 19:24:09 浏览: 18
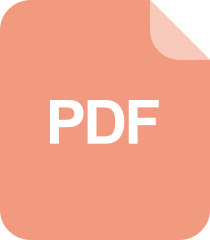
Python中常用操作字符串的函数与方法总结

在Python中,可以使用内置的`enumerate()`函数结合字符串切片来获取每个字符及其在字符串中的索引。这里是一个简单的示例程序:
```python
def find_char_positions(user_input):
# 检查用户输入是否为字符串
if not isinstance(user_input, str):
print("请输入一个字符串。")
return
positions = {}
for index, char in enumerate(user_input):
positions[char] = index + 1 # 字符串索引是从0开始的,加1得到实际位置
# 输出每个字符及其位置
for char, position in positions.items():
print(f"字符 '{char}' 的位置是 {position}")
# 获取用户输入
user_input = input("请输入一个字符串: ")
find_char_positions(user_input)
```
当你运行这个程序并输入字符串后,它会显示每个字符及其在输入字符串中的位置。
阅读全文
相关推荐
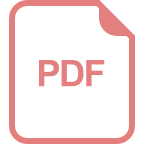
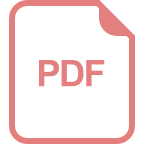















