从键盘输入字符,以“\n”结束,统计输入的数字0~9、空白符、和其他字符的个数
时间: 2024-10-26 19:02:50 浏览: 13
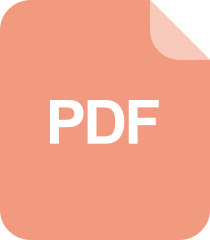
C语言统计一串字符中空格键、Tab键、回车键、字母、数字及其他字符的个数(Ctrl+Z终止输入)
要完成这个任务,你可以使用C++标准库中的`cin`和`std::string`。首先,你需要创建一个循环来接收用户的输入,直到遇到换行符`\n`。然后,我们可以遍历输入的字符串,用条件语句来统计不同类型的字符。
以下是一个简单的C++程序示例:
```cpp
#include <iostream>
#include <cctype> // 包含处理字符类型的方法
int main() {
std::string input;
int num_digits = 0, num_spaces = 0, other_chars = 0;
// 循环接收用户输入,直到遇到'\n'
while (true) {
char ch = getchar(); // 使用getchar获取并读取一个字符
if (ch == '\n') break; // 当遇到换行符时退出循环
// 检查字符类型并计数
if (isdigit(ch)) { // 如果是数字0-9
num_digits++;
} else if (isspace(ch)) { // 如果是空格或制表符
num_spaces++;
} else { // 其他非数字非空格字符
other_chars++;
}
}
// 输出结果
std::cout << "Number of digits: " << num_digits << "\n";
std::cout << "Number of spaces and tabs: " << num_spaces << "\n";
std::cout << "Number of other characters: " << other_chars << "\n";
return 0;
}
```
在这个程序中,`isdigit`函数用于检查字符是否为数字,`isspace`函数用于判断字符是否为空白(包括空格和制表符)。`getchar`函数从标准输入流读取一个字符,当遇到换行符时,我们跳出循环。
运行此程序后,它会等待用户输入,当你按下回车键后,它会统计输入的数字、空白符以及其他的字符数量。
阅读全文
相关推荐
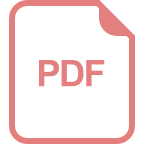
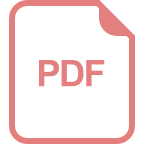















