从键盘输入字符,以“\n”结束,统计输入的数字0~9、空白符、和其他字符的个数,用字符数组的知识
时间: 2024-10-25 16:15:20 浏览: 30
要实现这个功能,你可以使用 C++ 的标准库 `std::cin` 来获取用户的输入,并遍历字符串来计数不同类型的字符。这里我们可以创建一个字符数组,同时定义三个变量分别用于存储0-9、空白符(包括空格和换行符)和其他字符的数量。
以下是一个简单的示例程序:
```cpp
#include <iostream>
#include <cctype> // 使用 isspace() 和 isdigit()
int countDigits(char input);
int countSpaces(char input);
int countOtherChars(char input);
int main() {
const int MAX_INPUT = 100; // 输入的最大长度
char input[MAX_INPUT];
std::cout << "请输入字符,以'\n'结束:";
std::cin.getline(input, MAX_INPUT); // 获取用户输入直到遇到'\n'
int numDigits = countDigits(input);
int numSpaces = countSpaces(input);
int numOtherChars = countOtherChars(input) - numSpaces; // 减去空白字符的数量
std::cout << "0-9字符数量: " << numDigits << "\n";
std::cout << "空白字符数量: " << numSpaces << "\n";
std::cout << "其他字符数量: " << numOtherChars << "\n";
return 0;
}
// 计算字符是否为数字
int countDigits(char input) {
int count = 0;
for (char c : input) {
if (isdigit(c)) {
count++;
}
}
return count;
}
// 计算字符是否为空白
int countSpaces(char input) {
int count = 0;
for (char c : input) {
if (isspace(c)) {
count++;
}
}
return count;
}
// 计算非数字和空白的其他字符
int countOtherChars(char input) {
int count = 0;
for (char c : input) {
if (!isdigit(c) && !isspace(c)) {
count++;
}
}
return count;
}
```
在这个程序中,我们定义了三个辅助函数 `countDigits()`、`countSpaces()` 和 `countOtherChars()` 分别用于计算数字、空白符和其它字符的个数。在 `main()` 函数里,我们首先读取用户输入,然后调用这些函数并输出结果。
阅读全文
相关推荐
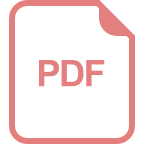
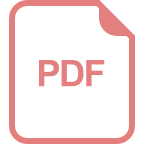
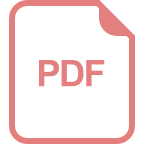















