用C语言写一个监控1000个bool量触发的次数,并把次数最多的前10个排序出来的程序
时间: 2024-10-05 17:02:30 浏览: 25
在C语言中编写这样的程序需要使用数组来存储1000个布尔值(bool量),并配合计数结构来跟踪每个值被触发的次数。这里我们假设你有一个名为`bool_array`的数组来存储状态,然后使用一个`struct`来记录每个元素及其对应的计数。最后,我们可以使用快速选择算法(QuickSelect)来找到次数最多的前10个元素,因为数组已经排好序了。
```c
#include <stdio.h>
#include <stdlib.h>
// 结构体用于存储bool值和计数
typedef struct {
bool value;
int count;
} BoolCount;
// 比较函数用于快速选择
int compare(const void *a, const void *b) {
return ((BoolCount *) a)->count - ((BoolCount *) b)->count;
}
void update_count(BoolCount bools[], int n) {
for (int i = 0; i < n; ++i) {
if (bools[i].value)
++bools[i].count;
}
}
void print_top_10(BoolCount bools[], int n) {
qsort(bools, n, sizeof(BoolCount), compare);
printf("Top 10 most triggered bool values:\n");
for (int i = 0; i < 10 && i < n; ++i) {
printf("%d: %d times\n", i + 1, bools[i].count);
}
}
int main() {
BoolCount bools[1000];
// 初始化或读取bool值
for (int i = 0; i < 1000; ++i) {
bools[i].value = ...; // 根据实际情况设置初始值
}
update_count(bools, 1000);
print_top_10(bools, 1000);
return 0;
}
```
阅读全文
相关推荐
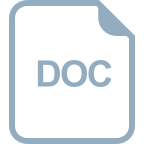
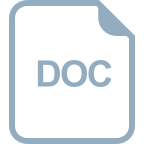
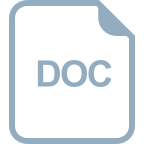



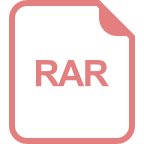











