1、定义单链表。 1)创建单链表; 2)插入操作; 3)删除操作; 4)存取操作; 5)查找操作。用C++写
时间: 2024-11-06 07:20:59 浏览: 6
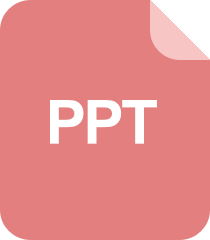
线性表操作在单链表的实现-链表的代码
1. 定义单链表:
单链表是一种线性数据结构,每个节点包含两个部分:数据域用于存储元素值,指针域用于指向下一个节点。在C++中,我们可以这样定义一个简单的单链表节点:
```cpp
struct ListNode {
int data; // 节点的数据
ListNode* next; // 指向下一个节点的指针
};
```
2. 创建单链表 (初始化一个空链表):
```cpp
ListNode* createList() {
return nullptr; // 初始链表为空,返回空指针
}
```
3. 插入操作 (在链表尾部添加新节点):
```cpp
void insertNode(ListNode*& head, int value) {
if (!head) {
head = new ListNode{value, nullptr}; // 如果链表为空,新建一个头节点
} else {
ListNode* current = head;
while (current->next != nullptr) {
current = current->next;
}
current->next = new ListNode{value, nullptr}; // 否则,在最后一个节点之后添加
}
}
```
4. 删除操作 (根据值或位置删除节点):
- 删除指定值的节点:
```cpp
void deleteNode(ListNode*& head, int value) {
ListNode* prev = nullptr, *current = head;
while (current != nullptr && current->data != value) {
prev = current;
current = current->next;
}
if (current != nullptr) {
if (prev == nullptr) { // 如果是头节点
head = current->next;
} else {
prev->next = current->next;
}
delete current; // 删除节点
}
}
```
- 删除特定位置的节点 (这里假设有一个函数如`findIndex`找到节点的位置):
```cpp
void deleteNodeByIndex(ListNode*& head, int index) {
if (index < 0 || !head) return;
for (int i = 0; i < index && head; ++i, head = head->next);
if (head) {
ListNode* temp = head->next;
head->next = head->next->next;
delete temp;
}
}
```
5. 存取操作 (获取链表中的某个节点值):
```cpp
int getValue(const ListNode* node) {
if (node != nullptr) {
return node->data;
} else {
throw std::runtime_error("Node is null");
}
}
```
6. 查找操作 (寻找链表中是否存在某个值):
```cpp
bool findValue(const ListNode* head, int value) {
ListNode* current = head;
while (current != nullptr) {
if (current->data == value) {
return true;
}
current = current->next;
}
return false;
}
```
阅读全文
相关推荐
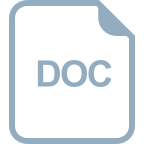
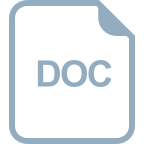

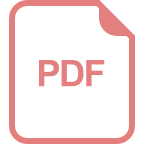
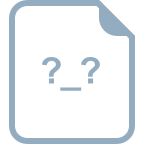
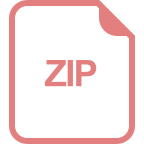
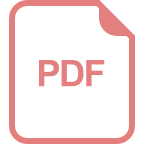
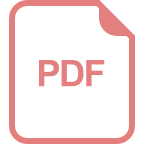
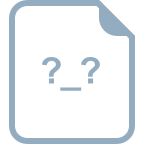
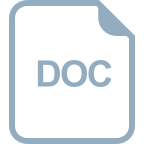
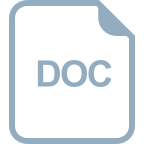
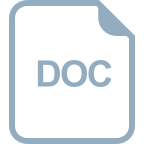
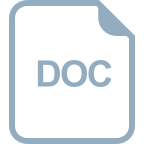
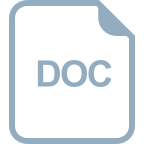
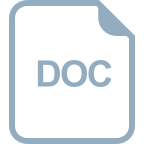
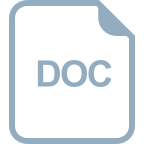
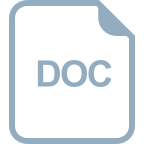
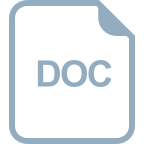