16位串行乘法器verilog
时间: 2025-01-04 10:23:09 浏览: 7
### 16-Bit Serial Multiplier Implementation in Verilog
A 16-bit serial multiplier can be implemented using shift and add operations. The design involves taking two 16-bit inputs, multiplying them bit by bit over multiple cycles to produce a final result.
Below is an example of how such a multiplier might look:
#### Module Definition
The module takes three ports: `clk` for clock signal, `reset_n` for active-low reset, and `start` to initiate multiplication. Two 16-bit vectors serve as operands (`a`, `b`) while another vector holds the product (`product`). A status flag indicates when computation completes.
```verilog
module serial_multiplier (
input wire clk,
input wire reset_n,
input wire start,
input wire [15:0] a,
input wire [15:0] b,
output reg [31:0] product,
output reg done
);
```
#### Internal Signals Declaration
Several internal signals manage state transitions during operation including counters tracking progress through bits being processed along with temporary storage variables used within calculations themselves.
```verilog
reg [4:0] count;
reg [15:0] multiplicand_reg;
reg [31:0] partial_product;
wire last_bit;
assign last_bit = (count == 5'd15); // Check if we're on the last iteration
```
#### State Machine Logic
This section outlines behavior based upon current conditions like whether starting up or processing individual stages until completion whereupon results become available externally via outputs defined earlier above under "Module Definition".
```verilog
always @(posedge clk or negedge reset_n) begin
if (!reset_n) begin
count <= 0;
multiplicand_reg <= 0;
partial_product <= 0;
product <= 0;
done <= 0;
end else if (start && !done) begin
case(count)
5'b00000 : begin
multiplicand_reg <= a;
partial_product <= {16'b0,b};
count <= count + 1;
end
default : begin
if(partial_product[0])
partial_product <= {partial_product[31], partial_product[30:1]} + {{16{multiplicand_reg[15]}}, multiplicand_reg};
else
partial_product <= {partial_product[31], partial_product[30:1]};
if(last_bit) begin
product <= partial_product;
done <= 1;
end
count <= count + 1;
multiplicand_reg <= {multiplicand_reg[14:0], 1'b0};
end
endcase
end
end
```
阅读全文
相关推荐





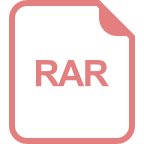






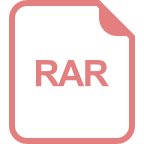




