利用c++编写一段关于学生信息的单链表并能插入和删除
时间: 2024-09-26 13:02:43 浏览: 23
在C++中,我们可以使用结构体(struct)和指针来创建一个简单的单链表来存储学生信息。首先,我们定义一个`Student`结构体,包含姓名和学号:
```cpp
#include <iostream>
using namespace std;
// 定义学生信息结构体
struct Student {
string name;
int id;
// 指向下一个节点的指针
struct Student* next;
};
// 插入节点函数
void insert(Student** head, const string& name, int id) {
// 创建新节点
Student* newNode = new Student();
newNode->name = name;
newNode->id = id;
newNode->next = nullptr; // 新节点默认没有下一个
// 如果链表为空,新节点成为头节点
if (*head == nullptr) {
*head = newNode;
return;
}
// 否则遍历到最后一个节点并添加新节点
Student* current = *head;
while (current->next != nullptr) {
current = current->next;
}
current->next = newNode;
}
// 删除节点函数(这里仅删除指定ID的学生)
void deleteNodeById(Student** head, int id) {
if (*head == nullptr) {
return;
}
// 遍历链表查找目标节点
Student* current = *head;
Student* prev = nullptr;
while (current != nullptr && current->id != id) {
prev = current;
current = current->next;
}
// 找到了就删除它
if (current != nullptr) {
if (prev == nullptr) { // 如果是头节点
*head = current->next;
} else {
prev->next = current->next;
}
delete current; // 释放内存
}
}
int main() {
// 初始化链表
Student* head = nullptr;
// 插入示例
insert(&head, "张三", 1001);
insert(&head, "李四", 1002);
// 输出链表
cout << "学生信息链表:\n";
Student* temp = head;
while (temp != nullptr) {
cout << temp->name << ", ID: " << temp->id << endl;
temp = temp->next;
}
// 删除示例
deleteNodeById(&head, 1001); // 删除ID为1001的学生
// 再次输出链表
cout << "\n删除操作后的链表:\n";
temp = head;
while (temp != nullptr) {
cout << temp->name << ", ID: " << temp->id << endl;
temp = temp->next;
}
return 0;
}
```
相关推荐
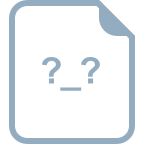
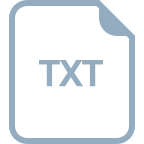
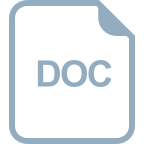














