用c++实现单链表的创建插入输出删除
时间: 2024-09-26 19:14:20 浏览: 11
在C++中,我们可以使用结构体或类来表示单链表。首先,我们创建一个节点结构体,包含数据域和指向下一个节点的指针:
```cpp
struct Node {
int data;
Node* next;
};
```
接下来,我们可以定义一个单链表类,包含插入、输出(遍历)和删除操作:
```cpp
class LinkedList {
private:
Node* head; // 链表头指针
public:
// 构造函数,初始化空链表
LinkedList() : head(nullptr) {}
// 插入节点到链表头部
void insert(int value) {
Node* newNode = new Node();
newNode->data = value;
newNode->next = head;
head = newNode;
}
// 输出链表中的所有元素(递归)
void printList() {
if (head == nullptr) {
cout << "链表为空" << endl;
return;
}
cout << head->data << " -> ";
printList(head->next);
}
// 删除指定值的节点(仅删除头节点)
void remove(int value) {
if (head && head->data == value) {
Node* temp = head;
head = head->next;
delete temp;
} else {
Node* current = head;
while (current && current->data != value) {
current = current->next;
}
if (current) {
Node* temp = current->next;
delete current;
current = temp;
}
}
}
};
```
相关推荐
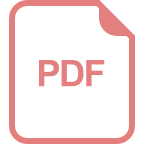
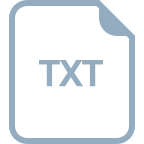
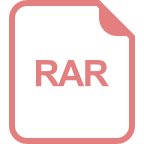
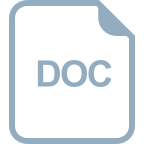
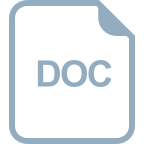
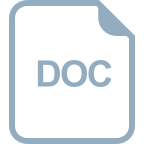
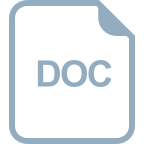
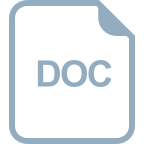
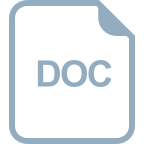
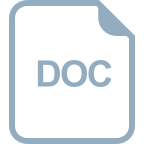
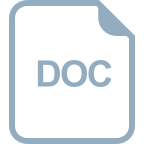
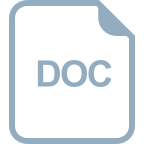





