建立单链表查找插入删除元素
时间: 2024-09-28 17:07:48 浏览: 46
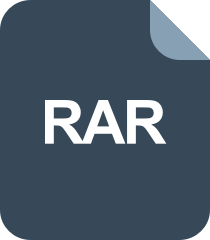
单链表的建立、插入、删除、查找.rar_单链表的建立、插入、删除、查找_插入删除

单链表是一种常见的数据结构,它由一系列节点组成,每个节点包含一个数据元素和一个指向下一个节点的指针。单链表的操作包括查找、插入和删除元素。
1. 查找元素:从链表的头节点开始,依次遍历每个节点,直到找到目标元素或者遍历到链表的末尾。如果找到目标元素,则返回该节点的指针;否则返回空指针。
2. 插入元素:在链表中插入一个新元素,需要先创建一个新节点,并将新节点的指针指向原来的下一个节点。然后将前一个节点的指针指向新节点即可。
3. 删除元素:从链表中删除一个元素,需要先找到该元素所在的节点,并将前一个节点的指针指向该节点的下一个节点。然后释放该节点的内存空间即可。
下面是一个简单的示例代码,实现了单链表的基本操作:
```c++
#include <iostream>
using namespace std;
// 定义单链表节点结构体
struct ListNode {
int val;
ListNode* next;
ListNode(int x) : val(x), next(NULL) {}
};
// 查找元素
ListNode* searchList(ListNode* head, int target) {
ListNode* cur = head;
while (cur != NULL && cur->val != target) {
cur = cur->next;
}
return cur;
}
// 插入元素
void insertList(ListNode* head, int val) {
ListNode* newNode = new ListNode(val);
newNode->next = head->next;
head->next = newNode;
}
// 删除元素
void deleteList(ListNode* head, int val) {
ListNode* cur = head;
while (cur->next != NULL && cur->next->val != val) {
cur = cur->next;
}
if (cur->next != NULL) {
ListNode* temp = cur->next;
cur->next = temp->next;
delete temp;
}
}
int main() {
// 创建链表
ListNode* head = new ListNode(0);
insertList(head, 1);
insertList(head, 2);
insertList(head, 3);
// 查找元素
ListNode* node = searchList(head, 2);
if (node != NULL) {
cout << "Found: " << node->val << endl;
} else {
cout << "Not found" << endl;
}
// 删除元素
deleteList(head, 2);
// 输出链表
ListNode* cur = head->next;
while (cur != NULL) {
cout << cur->val << " ";
cur = cur->next;
}
cout << endl;
return 0;
}
```
阅读全文
相关推荐
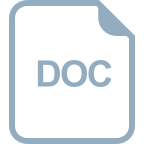






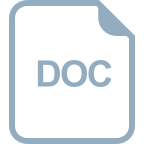
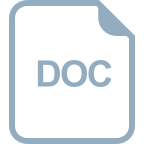






