.编写程序对线性表的元素实现链式存储,包括创建单链表、在单链表上插入、删除、查找数据,并能输出单链表中所有元素及销毁单链表等; 2.对线性表的每个基本操作用单独的函数实现;
时间: 2024-09-29 14:05:50 浏览: 33
在编程中,特别是使用面向对象的语言如C++或Python,我们可以创建一个名为`ListNode`或`Node`的类来表示单链表的节点,包含一个数据域以及一个指向下一个节点的指针。以下是针对这个需求的一些关键步骤:
1. **创建单链表**:
```python
class SinglyLinkedList:
def __init__(self):
self.head = None
```
这里定义了一个简单的链表类,初始化时链表为空。
2. **插入元素**:
```python
def insert_at_start(self, data):
new_node = ListNode(data)
new_node.next = self.head
self.head = new_node
def insert_at_end(self, data):
if not self.head:
self.head = ListNode(data)
return
current = self.head
while current.next:
current = current.next
current.next = ListNode(data)
```
分别实现了在开始和末尾插入新节点的功能。
3. **删除元素**:
```python
def delete_node(self, key):
if self.head is None or self.head.data == key:
self.head = self.head.next
return
current = self.head
while current.next and current.next.data != key:
current = current.next
if current.next is not None:
current.next = current.next.next
```
这里有删除首部节点和删除特定值节点的方法。
4. **查找数据**:
```python
def search(self, key):
current = self.head
while current:
if current.data == key:
return True
current = current.next
return False
```
此方法用于判断链表中是否存在某个值。
5. **遍历并打印元素**:
```python
def print_list(self):
elements = []
current = self.head
while current:
elements.append(current.data)
current = current.next
print(elements)
6. **销毁单链表**:
这通常是自动处理的,当不再需要链表时,它的内存会随着引用的消失而被垃圾回收机制释放。如果要手动清理,可以设置`head`为`None`。
阅读全文
相关推荐
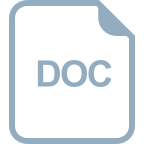
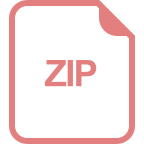
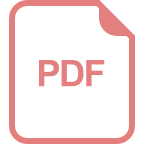




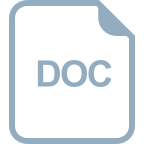
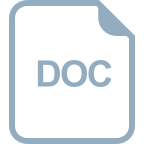
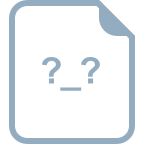
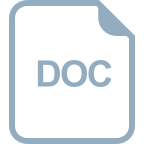
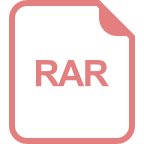
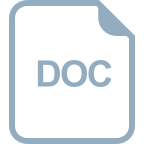
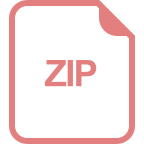
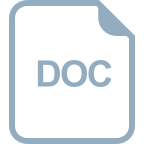
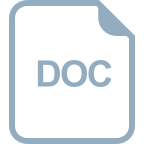
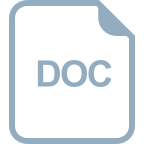
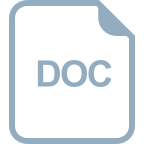
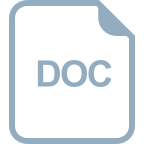
