使用dev c++编写(1)创建一个带头结点的单链表。 要求:此单链表长度为10;创建完成后,输出显示所创建单链表各结点的值。 (2)实现单链表的插入,并显示完成插入后单链表各结点的值。 要求:插入一个新的结点,其值为27,成为单链表的第5个结点;插入完成后,输出显示此时单链表的长度以及各结点的值。 (3)实现单链表的删除,完成删除后,输出单链表各结点的值。 (4)编程实现单链表的查找。\
时间: 2024-02-03 20:13:33 浏览: 64
以下是使用 dev c++编写的代码实现:
```c++
#include <stdio.h>
#include <stdlib.h>
struct Node {
int value;
struct Node* next;
};
struct Node* createLinkedList(int n) {
struct Node* head = (struct Node*)malloc(sizeof(struct Node));
head->next = NULL;
struct Node* p = head;
for (int i = 1; i <= n; i++) {
struct Node* node = (struct Node*)malloc(sizeof(struct Node));
node->value = i;
node->next = NULL;
p->next = node;
p = node;
}
return head;
}
void printLinkedList(struct Node* head) {
struct Node* p = head->next;
while (p != NULL) {
printf("%d ", p->value);
p = p->next;
}
printf("\n");
}
void insertNode(struct Node* head, int value, int index) {
struct Node* p = head;
for (int i = 0; i < index - 1; i++) {
p = p->next;
}
struct Node* node = (struct Node*)malloc(sizeof(struct Node));
node->value = value;
node->next = p->next;
p->next = node;
}
void deleteNode(struct Node* head, int index) {
struct Node* p = head;
for (int i = 0; i < index - 1; i++) {
p = p->next;
}
struct Node* q = p->next;
p->next = q->next;
free(q);
}
struct Node* findNode(struct Node* head, int value) {
struct Node* p = head->next;
while (p != NULL && p->value != value) {
p = p->next;
}
return p;
}
int main() {
struct Node* head = createLinkedList(10);
printf("创建单链表:\n");
printLinkedList(head);
insertNode(head, 27, 5);
printf("插入新结点:\n");
printLinkedList(head);
deleteNode(head, 5);
printf("删除结点:\n");
printLinkedList(head);
struct Node* node = findNode(head, 7);
if (node != NULL) {
printf("查找到结点值为%d\n", node->value);
} else {
printf("未找到结点\n");
}
return 0;
}
```
其中,`createLinkedList`函数用于创建一个带头结点的单链表,参数 `n` 表示链表长度;`printLinkedList`函数用于输出链表的所有结点的值;`insertNode`函数用于在链表的第 `index` 个位置插入一个值为 `value` 的新结点;`deleteNode`函数用于删除链表的第 `index` 个结点;`findNode`函数用于查找链表中值为 `value` 的结点,返回该结点的指针。在 `main` 函数中,按照题目要求调用以上函数,完成相应的操作。
阅读全文
相关推荐
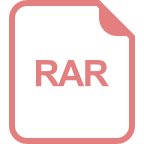
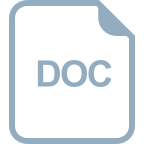
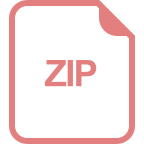















