c++ vector二维数组,分别获取长和宽
时间: 2024-07-22 17:01:17 浏览: 102
在 C++ 中,如果你有一个 `std::vector<std::vector<T>>` 类型的二维向量,其中 `T` 是任意类型的元素,想要获取这个二维向量的长度(即行数)和宽度(即列数),你可以这样做:
```cpp
#include <vector>
using namespace std;
int main() {
// 假设你有如下的二维向量
vector<vector<int>> myVector = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
int rows = myVector.size(); // 获取行数(二维向量的大小)
int cols = myVector.size(); // 获取列数,这里假设所有行都有相同的列数
cout << "行数: " << rows << endl;
cout << "列数: " << cols << endl;
return 0;
}
```
在这个例子中,`myVector.size()` 返回整个向量的行数,而 `myVector.size()` 则返回第一行(假设其他行也有相同数量的元素)的列数,因为 C++ 的 `std::vector` 默认是动态调整大小,所以需要检查实际的第一行确定列数。
相关问题
c++vector二维数组
C++中的vector是一个动态数组容器,可以方便地进行元素的插入、删除和访问。二维数组可以通过vector嵌套来实现。
下面是使用vector实现二维数组的示例代码:
```cpp
#include <iostream>
#include <vector>
int main() {
// 创建一个二维数组,大小为3行4列
std::vector<std::vector<int>> matrix(3, std::vector<int>(4));
// 给二维数组赋值
for (int i = 0; i < matrix.size(); i++) {
for (int j = 0; j < matrix[i].size(); j++) {
matrix[i][j] = i * matrix[i].size() + j;
}
}
// 输出二维数组的值
for (int i = 0; i < matrix.size(); i++) {
for (int j = 0; j < matrix[i].size(); j++) {
std::cout << matrix[i][j] << " ";
}
std::cout << std::endl;
}
return 0;
}
```
上述代码中,我们首先创建了一个大小为3行4列的二维数组`matrix`,其中每个元素都是int类型。然后使用两层循环给二维数组赋值,并输出二维数组的值。
c++ vector 二维数组
要创建一个二维vector数组c,你可以使用以下方法:
1. 使用vector的构造函数初始化二维数组c,并指定初始值为0。例如,使用以下代码可以创建一个行数为row,列数为column的二维数组,并将所有元素初始化为0:
vector<vector<int>> c(row, vector<int>(column, 0));
2. 使用push_back()函数逐行添加向量。例如,使用以下代码可以创建一个空的二维数组c,并逐行添加向量:
vector<vector<int>> c;
vector<int> row1;
row1.push_back(0);
row1.push_back(1);
row1.push_back(2);
c.push_back(row1);
vector<int> row2;
row2.push_back(3);
row2.push_back(4);
row2.push_back(5);
c.push_back(row2);
3. 使用索引方式直接操作二维数组。例如,使用以下代码可以创建一个空的二维数组c,并直接通过索引方式添加元素:
vector<vector<int>> c;
c.push_back(0);
c.push_back(1);
c.push_back(2);
c.push_back(3);
c.push_back(4);
c.push_back(5);
请注意,在使用索引方式操作二维数组时,确保已经为每一行创建了向量。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *3* [c++---vector二维数组](https://blog.csdn.net/m0_50046535/article/details/125344884)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
- *2* [C++--vector数组使用](https://blog.csdn.net/JUST__Tw/article/details/112221317)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
相关推荐
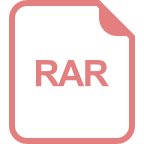
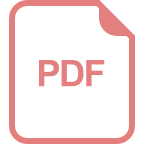
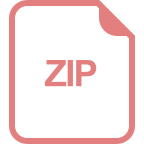












