利用c++语言写一个实现贪心算法的导航算法应用
时间: 2024-10-20 21:14:12 浏览: 16
在C++中,编写一个简单的贪婪算法用于导航通常会涉及路径规划的问题,比如在一个有障碍物的地图上找到从起点到终点的最短路径。这里我们可以用Dijkstra算法作为基础,因为它也是一种贪心策略,它总是选择当前看起来最优的未访问节点。
```cpp
#include <iostream>
#include <vector>
#include <queue>
#include <climits>
// 定义地图数据结构
struct Node {
int x, y;
int cost; // 节点成本,假设越小越好(即距离)
};
// 使用优先队列存储待处理节点
class PriorityQueue {
public:
std::vector<Node> queue_;
bool empty() const { return queue_.empty(); }
void push(Node n) { queue_.push_back(n); }
Node pop() {
if (queue_.empty()) return Node{INT_MAX, INT_MAX};
return queue_[0];
}
};
// 贪婪导航函数
void greedyNavigation(const std::vector<Node>& graph, Node start, Node end) {
PriorityQueue pq;
pq.push(start);
start.cost = 0;
while (!pq.empty()) {
Node current = pq.pop();
if (current.x == end.x && current.y == end.y) {
std::cout << "Path found with cost: " << current.cost << std::endl;
break;
}
for (const Node& neighbor : graph) {
int newCost = current.cost + neighbor.cost;
if (newCost < neighbor.cost) {
neighbor.cost = newCost;
pq.push(neighbor);
}
}
}
}
int main() {
// 假设我们有一个二维网格表示地图,其中值为0表示可以通行,其他值为障碍
std::vector<Node> graph; // 初始化地图在这里
Node start = {0, 0}; // 起点坐标
Node end = {graph.size() - 1, graph[0].y}; // 终点坐标
greedyNavigation(graph, start, end);
return 0;
}
阅读全文
相关推荐
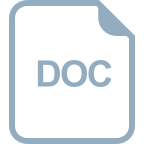
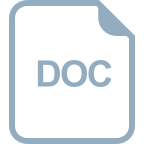
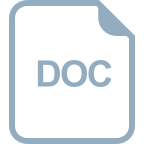
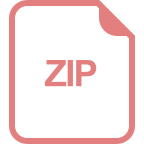
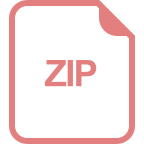
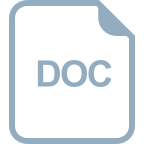
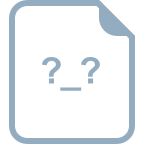
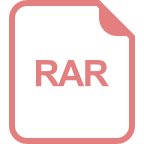
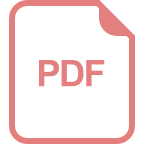
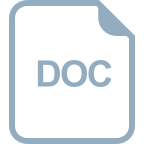
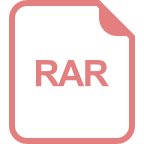
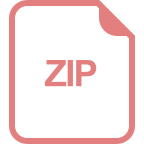
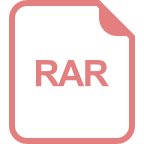
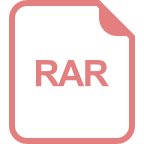
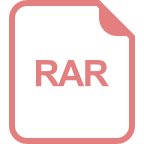