用python编写一个程序,要求如下: 1、建立一个窗口,窗口里包含:10个选项标签、一个确定按钮、一个退出按钮。 2、窗口里有背景图片和背景音乐。 3、选择一个选项标签并单击确定按钮后,跳转到另一个python程序并运行。
时间: 2024-09-23 18:07:33 浏览: 15
在Python中,你可以使用`tkinter`库创建一个简单的图形用户界面(GUI),同时结合`pygame`库来处理音效和图像。以下是一个简化的示例代码:
```python
import tkinter as tk
from tkinter import ttk
import pygame
# 初始化Pygame
pygame.init()
# 设置背景音乐
background_music = pygame.mixer.music.load('path_to_your_music.mp3')
pygame.mixer.music.play(-1) # -1表示无限循环播放
class Application(tk.Tk):
def __init__(self):
super().__init__()
self.title("Option Selection")
self.geometry("400x300")
# 背景图片
bg_img_path = 'path_to_your_image.jpg'
photo = tk.PhotoImage(file=bg_img_path)
label_back = tk.Label(self, image=photo, bg="white", compound=tk.CENTER)
label_back.pack(fill='both', expand=True)
# 选项标签和按钮
options = ['Option 1', 'Option 2', 'Option 3', ...] # 根据需要填充选项
self.labels = []
for i, option in enumerate(options):
frame = ttk.Frame(self)
label = ttk.Label(frame, text=option, anchor='center')
label.pack()
self.labels.append(label)
frame.grid(row=i // 5, column=i % 5)
self.quit_button = tk.Button(self, text="Exit", command=self.destroy)
self.quit_button.pack(side="bottom")
self.determine_button = tk.Button(self, text="Determine", command=self.select_option)
self.determine_button.pack(side="bottom")
def select_option(self):
selected_index = int(self.labels[-1]["cursor"][0]) # 获取鼠标点击位置对应的索引
# 这里只是一个例子,你需要替换为实际的另一个程序路径和运行逻辑
self.withdraw() # 隐藏当前窗口
new_program_path = "path_to_other_program.py"
exec(open(new_program_path).read()) # 使用exec打开并运行新程序
if __name__ == "__main__":
app = Application()
app.mainloop()
相关推荐
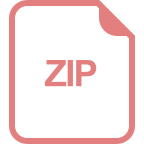
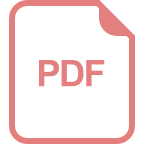
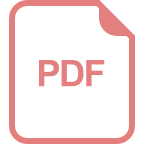














