用pycharm写一元二次方程的图像及其切线动画
时间: 2024-10-10 21:03:26 浏览: 41
在PyCharm中编写一个一元二次方程的图像及其切线动画,你可以遵循以下步骤:
1. **导入必要的库**:
首先,你需要import matplotlib.pyplot (用于绘制图形) 和 numpy (用于数值计算)。
```python
import numpy as np
import matplotlib.pyplot as plt
from matplotlib.animation import FuncAnimation
```
2. **定义一元二次函数**:
设定一个通用的一元二次方程,例如`ax^2 + bx + c = 0`。用户可以输入系数a、b、c。
```python
def quadratic_function(a=1, b=0, c=-1):
def equation(x):
return a * x**2 + b * x + c
return equation
```
3. **生成x值和y值**:
创建一个x值范围,并计算对应的y值。
```python
def create_data(equation, x_range=(-10, 10, 0.01)):
x = np.arange(*x_range)
y = equation(x)
return x, y
```
4. **创建基础图形**:
使用matplotlib画出一元二次曲线。
```python
fig, ax = plt.subplots()
line, = ax.plot([], [], lw=2)
def init():
line.set_data([], [])
return line,
def animate(i):
x, y = create_data(quadratic_function())
line.set_data(x[:i], y[:i])
return line,
```
5. **添加切线动画**:
计算每个点的导数,表示切线斜率,并在动画中加入切线。
```python
def derivative(equation, x):
return 2 * a * x + b
def add_tangent(line, i, ax):
x, y = create_data(quadratic_function(), (-10, x[i] - 0.1, 0.001))
dx = x[1] - x[0]
tangent_y = derivative(equation, x[i]) * dx + y[i]
slope, intercept = tangent_y - y[i], -derivative(equation, x[i]) * x[i]
tangent_line, = ax.plot([x[i], x[i]], [y[i], tangent_y], 'r--')
return line, tangent_line
ani = FuncAnimation(fig, animate, frames=len(create_data(quadratic_function())[0]),
init_func=init, blit=True, interval=50, repeat=True,
fargs=(add_tangent, ax))
plt.show()
阅读全文
相关推荐
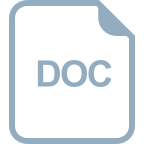
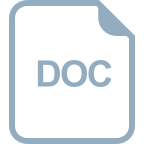
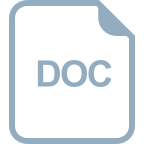





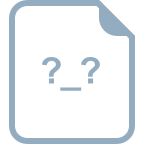









