购物车vue项目代码
时间: 2023-11-17 09:08:04 浏览: 138
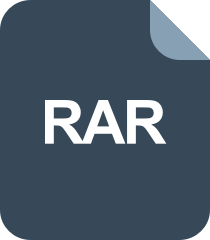
购物车项目代码

物车Vue项目代码需要实现以下功能:
1. 商品列表展示
2. 加入购物车功能
3. 购物车展示
4. 购物车商品数量修改
5. 购物车商品删除
6. 结算功能
以下是购物车Vue项目代码的实现步骤:
1. 创建Vue项目并安装Vue-router和Vuex插件
2. 创建商品列表组件List.vue,实现商品列表展示和加入购物车功能
3. 创建购物车组件Check.vue,实现购物车展示、商品数量修改和删除功能
4. 创建路由组件Product.vue和Home.vue,分别用于商品详情页和首页
5. 在App.vue中引入组件和路由,并设置路由路径
6. 创建Vuex store.js文件,实现购物车数据的存储和管理
7. 在base.scss中设置基础样式
以下是购物车Vue项目代码的完整代码:
(1) main.js
```
import Vue from 'vue'
import App from './App.vue'
import router from './router'
import store from './store'
Vue.config.productionTip = false
new Vue({
router,
store,
render: h => h(App)
}).$mount('#app')
```
(2) Router.js
```
import Vue from 'vue'
import Router from 'vue-router'
import Home from './views/Home.vue'
import Product from './views/Product.vue'
import Check from './views/Check.vue'
Vue.use(Router)
export default new Router({
routes: [
{
path: '/',
name: 'home',
component: Home
},
{
path: '/product/:id',
name: 'product',
component: Product
},
{
path: '/check',
name: 'check',
component: Check
}
]
})
```
(3) store.js
```
import Vue from 'vue'
import Vuex from 'vuex'
Vue.use(Vuex)
export default new Vuex.Store({
state: {
cart: []
},
mutations: {
addToCart(state, product) {
let item = state.cart.find(item => item.id === product.id)
if (item) {
item.quantity++
} else {
state.cart.push({
...product,
quantity: 1
})
}
},
removeFromCart(state, product) {
let index = state.cart.findIndex(item => item.id === product.id)
if (index !== -1) {
state.cart.splice(index, 1)
}
},
updateQuantity(state, payload) {
let item = state.cart.find(item => item.id === payload.id)
if (item) {
item.quantity = payload.quantity
}
}
},
actions: {
addToCart({ commit }, product) {
commit('addToCart', product)
},
removeFromCart({ commit }, product) {
commit('removeFromCart', product)
},
updateQuantity({ commit }, payload) {
commit('updateQuantity', payload)
}
},
getters: {
cartTotal(state) {
return state.cart.reduce((total, item) => total + item.price * item.quantity, 0)
}
}
})
```
(4) App.vue
```
<template>
<div id="app">
<router-view></router-view>
</div>
</template>
<script>
export default {
name: 'App'
}
</script>
<style lang="scss">
@import './assets/base.scss';
</style>
```
(5) List.vue
```
<template>
<div class="list">
<div class="item" v-for="product in products" :key="product.id">
<router-link :to="{ name: 'product', params: { id: product.id } }">
<img :src="product.image" alt="">
<h3>{{ product.name }}</h3>
<p>{{ product.price }}</p>
</router-link>
<button @click="addToCart(product)">加入购物车</button>
</div>
</div>
</template>
<script>
import { mapActions } from 'vuex'
export default {
name: 'List',
computed: {
products() {
return this.$store.state.products
}
},
methods: {
...mapActions(['addToCart']),
}
}
</script>
<style lang="scss">
.list {
display: flex;
flex-wrap: wrap;
justify-content: space-between;
.item {
width: 30%;
margin-bottom: 20px;
img {
width: 100%;
}
h3 {
margin-top: 10px;
font-size: 16px;
}
p {
margin-top: 5px;
font-size: 14px;
color: #f60;
}
button {
margin-top: 10px;
padding: 5px 10px;
border: none;
background-color: #f60;
color: #fff;
cursor: pointer;
}
}
}
</style>
```
(6) Home.vue
```
<template>
<div class="home">
<h2>商品列表</h2>
<List />
</div>
</template>
<script>
import List from '../components/List.vue'
export default {
name: 'Home',
components: {
List
}
}
</script>
<style lang="scss">
.home {
padding: 20px;
}
</style>
```
(7) Product.vue
```
<template>
<div class="product">
<img :src="product.image" alt="">
<h2>{{ product.name }}</h2>
<p>{{ product.price }}</p>
<button @click="addToCart(product)">加入购物车</button>
</div>
</template>
<script>
import { mapActions } from 'vuex'
export default {
name: 'Product',
computed: {
product() {
return this.$store.state.products.find(product => product.id === this.$route.params.id)
}
},
methods: {
...mapActions(['addToCart']),
}
}
</script>
<style lang="scss">
.product {
text-align: center;
img {
width: 50%;
}
h2 {
margin-top: 20px;
font-size: 24px;
}
p {
margin-top: 10px;
font-size: 20px;
color: #f60;
}
button {
margin-top: 20px;
padding: 10px 20px;
border: none;
background-color: #f60;
color: #fff;
cursor: pointer;
}
}
</style>
```
(8) Check.vue
```
<template>
<div class="check">
<h2>购物车</h2>
<div class="cart">
<div class="item" v-for="item in cart" :key="item.id">
<img :src="item.image" alt="">
<h3>{{ item.name }}</h3>
<p>{{ item.price }}</p>
<div class="quantity">
<button @click="updateQuantity(item.id, item.quantity - 1)">-</button>
<input type="number" v-model.number="item.quantity">
<button @click="updateQuantity(item.id, item.quantity + 1)">+</button>
</div>
<button @click="removeFromCart(item)">删除</button>
</div>
</div>
<div class="total">总价:{{ cartTotal }}</div>
</div>
</template>
<script>
import { mapState, mapActions } from 'vuex'
export default {
name: 'Check',
computed: {
...mapState(['cart', 'cartTotal'])
},
methods: {
...mapActions(['removeFromCart', 'updateQuantity'])
}
}
</script>
<style lang="scss">
.check {
padding: 20px;
.cart {
display: flex;
flex-wrap: wrap;
justify-content: space-between;
.item {
width: 30%;
margin-bottom: 20px;
img {
width: 100%;
}
h3 {
margin-top: 10px;
font-size: 16px;
}
p {
margin-top: 5px;
font-size: 14px;
color: #f60;
}
.quantity {
margin-top: 10px;
display: flex;
align-items: center;
button {
padding: 5px 10px;
border: none;
background-color: #f60;
color: #fff;
cursor: pointer;
}
input {
width: 50px;
text-align: center;
border: none;
border-bottom: 1px solid #ccc;
margin: 0 10px;
}
}
button {
margin-top: 10px;
padding: 5px 10px;
border: none;
background-color: #f60;
color: #fff;
cursor: pointer;
}
}
}
.total {
margin-top: 20px;
text-align: right;
font-size: 20px;
color: #f60;
}
}
</style>
```
(9) base.scss
```
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
body {
font-family: 'Helvetica Neue', Helvetica, Arial, sans-serif;
font-size: 16px;
color: #333;
}
a {
text-decoration: none;
color: #333;
}
ul {
list-style: none;
}
button {
outline: none;
}
input {
outline: none;
}
.container {
max-width: 1200px;
margin: 0 auto;
}
.clearfix::after {
content: '';
display: block;
clear: both;
}
.text-center {
text-align: center;
}
.mt-20 {
margin-top: 20px;
}
.mb-20 {
margin-bottom: 20px;
}
.p-20 {
padding: 20px;
}
.bg-f60 {
background-color: #f60;
color: #fff;
}
.flex {
display: flex;
align-items: center;
}
.flex-1 {
flex: 1;
}
@media (max-width: 768px) {
.container {
padding: 0 20px;
}
.list .item {
width: 48%;
}
.product img {
width: 80%;
}
.check .item {
width: 48%;
}
}
```
阅读全文
相关推荐
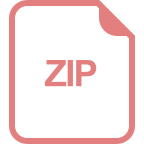
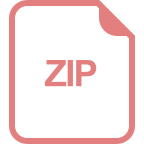
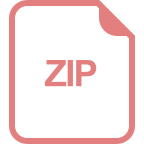
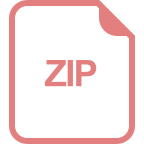
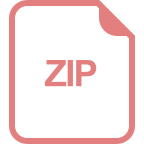
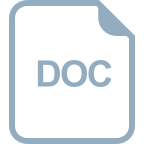
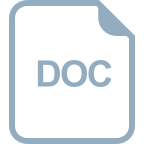
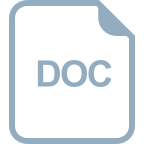
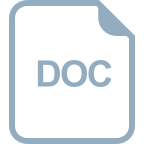
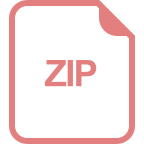
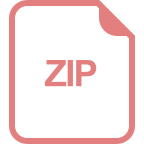
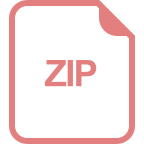
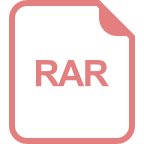
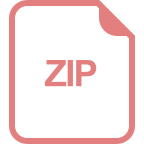