完整c语言井字棋代码
时间: 2024-12-18 14:15:50 浏览: 16
编写完整的C语言井字棋代码涉及到游戏循环、用户输入处理、游戏状态管理等多个部分。下面是一个简化的井字棋游戏的基本框架示例:
```c
#include <stdio.h>
#include <stdlib.h>
#define BOARD_SIZE 3
typedef struct {
char board[BOARD_SIZE][BOARD_SIZE];
int turn;
} TicTacToe;
void print_board(TicTacToe game) {
for (int i = 0; i < BOARD_SIZE; ++i) {
for (int j = 0; j < BOARD_SIZE; ++j) {
printf("%c ", game.board[i][j]);
}
printf("\n");
}
}
// 检查游戏是否结束并返回胜利者
char check_win(TicTacToe game) {
// ... (这里需要检查所有可能的获胜组合)
return 'X'; // 示例,只考虑了先手为胜的情况
}
// 主游戏循环
void play_game(TicTacToe *game) {
while (!check_win(*game)) {
if (*game->turn == 'X') {
int row, col;
printf("Player X, enter row and column (0-%d): ", BOARD_SIZE - 1);
scanf("%d %d", &row, &col);
game->board[row][col] = 'X';
} else {
// Player O's turn, same logic as above but with 'O'
}
print_board(*game);
game->turn = *game->turn == 'X' ? 'O' : 'X';
}
if (check_win(*game) == 'X') {
printf("Player X wins!\n");
} else {
printf("It's a tie!\n");
}
}
int main() {
TicTacToe game;
game.turn = 'X';
game.board = '\0'; // 初始化全为空
play_game(&game);
return 0;
}
```
这个例子只是一个基础版本,实际的完整代码会包括错误处理(如非法输入)、游戏结束条件的详细检查等。如果你想了解更复杂的实现,可以搜索"tic tac toe c++ source code"来找到更多详细的教程。
阅读全文
相关推荐
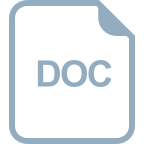
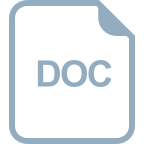
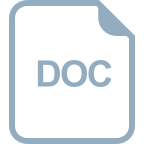

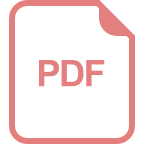
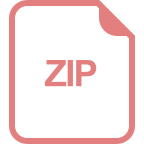





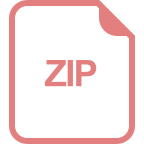
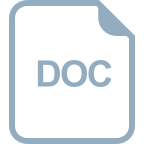

