用C 语言写单链表的倒置 L->1->2->3->4->NULL 倒置后: L >4->3->2->1->NULL 2.顺序表的创建/插入/删除|
时间: 2024-06-12 07:09:34 浏览: 99
单链表的倒置可以通过修改节点的指针来实现。以下是用C语言实现单链表倒置的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义节点结构
struct Node {
int data;
struct Node* next;
};
// 创建新节点
struct Node* createNode(int data) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = data;
newNode->next = NULL;
return newNode;
}
// 倒置单链表
struct Node* reverseList(struct Node* head) {
struct Node* prev = NULL;
struct Node* current = head;
struct Node* next = NULL;
while (current != NULL) {
next = current->next;
current->next = prev;
prev = current;
current = next;
}
return prev;
}
// 打印单链表
void printList(struct Node* head) {
struct Node* current = head;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
}
int main() {
// 创建示例链表
struct Node* L = createNode(1);
L->next = createNode(2);
L->next->next = createNode(3);
L->next->next->next = createNode(4);
printf("原始链表: ");
printList(L);
// 倒置链表
struct Node* reversedList = reverseList(L);
printf("\n倒置后的链表: ");
printList(reversedList);
return 0;
}
```
输出结果为:
原始链表: 1 2 3 4
倒置后的链表: 4 3 2 1
阅读全文
相关推荐












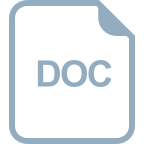


