用c语言写一个单链表的倒置方法
时间: 2024-05-15 20:18:58 浏览: 91
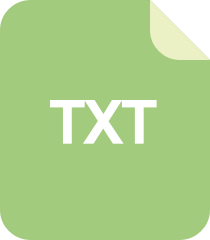
单链表的逆置(c语言)
好的,这个问题属于编程问题,我可以回答。以下是用C语言实现单链表倒置的方法:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点的结构体
typedef struct node {
int data;
struct node* next;
} Node, *pNode;
// 创建链表
pNode createList(int n) {
int i;
pNode head, p, q;
head = (pNode)malloc(sizeof(Node));
head->next = NULL;
q = head;
for (i = 0; i < n; i++) {
p = (pNode)malloc(sizeof(Node));
scanf("%d", &p->data);
p->next = NULL;
q->next = p;
q = p;
}
return head;
}
// 打印链表
void printList(pNode head) {
pNode p = head->next;
while (p != NULL) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
// 倒置链表
pNode reverseList(pNode head) {
pNode p, q, r;
p = head->next;
q = NULL;
while (p != NULL) {
r = p->next;
p->next = q;
q = p;
p = r;
}
head->next = q;
return head;
}
int main() {
int n;
pNode head, p, q;
printf("请输入节点个数n:");
scanf("%d", &n);
head = createList(n);
printf("原始列表:");
printList(head);
head = reverseList(head);
printf("倒置后的列表:");
printList(head);
return 0;
}
```
通过这段程序,可以输入一个单链表,并将其倒置后输出。
阅读全文
相关推荐








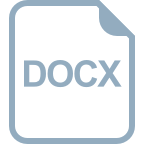




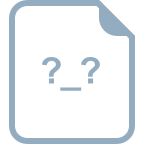
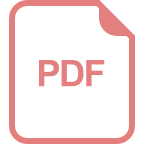
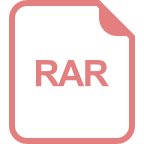