java sftp线程池下载文件
时间: 2024-03-27 08:33:38 浏览: 189
在Java中,可以使用SFTP(SSH File Transfer Protocol)协议来进行文件的下载。而线程池是一种用于管理和复用线程的机制,可以提高程序的性能和效率。下面是使用线程池进行SFTP文件下载的步骤:
1. 导入相关的依赖库:
```
import com.jcraft.jsch.ChannelSftp;
import com.jcraft.jsch.JSch;
import com.jcraft.jsch.Session;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
```
2. 创建一个线程池:
```
ExecutorService executor = Executors.newFixedThreadPool(5);
```
3. 定义一个任务类,实现Runnable接口:
```
public class SftpDownloadTask implements Runnable {
private String host;
private int port;
private String username;
private String password;
private String remoteFilePath;
private String localFilePath;
public SftpDownloadTask(String host, int port, String username, String password, String remoteFilePath, String localFilePath) {
this.host = host;
this.port = port;
this.username = username;
this.password = password;
this.remoteFilePath = remoteFilePath;
this.localFilePath = localFilePath;
}
@Override
public void run() {
try {
JSch jsch = new JSch();
Session session = jsch.getSession(username, host, port);
session.setPassword(password);
session.setConfig("StrictHostKeyChecking", "no");
session.connect();
ChannelSftp channelSftp = (ChannelSftp) session.openChannel("sftp");
channelSftp.connect();
channelSftp.get(remoteFilePath, localFilePath);
channelSftp.disconnect();
session.disconnect();
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
4. 提交任务到线程池:
```
String host = "your_host";
int port = 22;
String username = "your_username";
String password = "your_password";
String remoteFilePath = "/path/to/remote/file";
String localFilePath = "/path/to/local/file";
SftpDownloadTask task = new SftpDownloadTask(host, port, username, password, remoteFilePath, localFilePath);
executor.execute(task);
```
5. 关闭线程池:
```
executor.shutdown();
```
这样就可以使用线程池进行SFTP文件下载了。
阅读全文
相关推荐
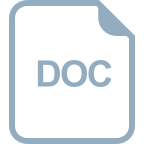
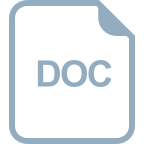
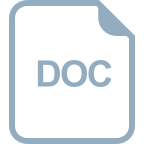
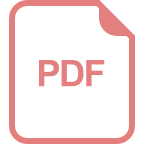
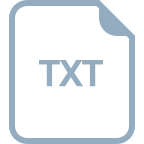
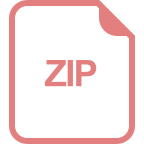
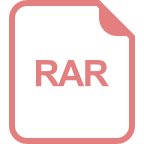
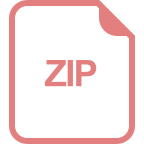
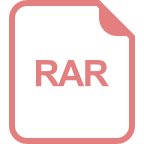
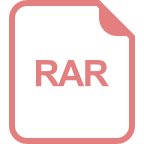
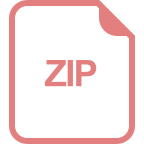
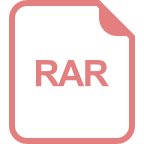
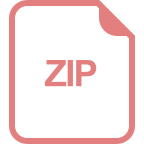
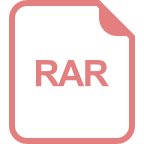
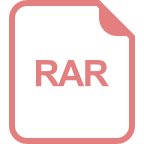
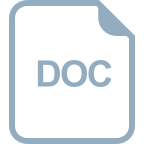
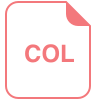
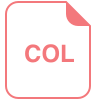
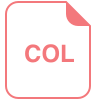