请根据文档内容提供VSCode代码,如需要添加图片等请标注出来
时间: 2024-12-12 22:30:44 浏览: 12
根据文档内容,《Web 前端设计与开发》期末综合实验要求开发一个预约挂号前端系统。以下是基于 Vue.js 和 ElementUI 的 VSCode 项目结构和关键代码示例。为了简洁起见,我将提供主要文件的内容,并标注需要添加图片或资源的地方。
### 项目结构
```
appointment-system/
├── public/
│ └── index.html
├── src/
│ ├── assets/
│ │ └── logo.png # 需要添加项目图标或其他静态资源
│ ├── components/
│ │ ├── DoctorCard.vue
│ │ ├── LoginForm.vue
│ │ ├── RegisterForm.vue
│ │ └── UserInformationForm.vue
│ ├── router/
│ │ └── index.js
│ ├── store/
│ │ └── index.js
│ ├── views/
│ │ ├── HomeView.vue
│ │ ├── LoginView.vue
│ │ ├── RegisterView.vue
│ │ ├── DoctorListView.vue
│ │ ├── AppointmentView.vue
│ │ └── HistoryView.vue
│ ├── App.vue
│ └── main.js
├── package.json
└── README.md
```
### 关键代码示例
#### `public/index.html`
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>预约挂号前端系统</title>
</head>
<body>
<div id="app"></div>
</body>
</html>
```
#### `src/main.js`
```javascript
import { createApp } from 'vue';
import App from './App.vue';
import router from './router';
import store from './store';
import ElementUI from 'element-ui';
import 'element-ui/lib/theme-chalk/index.css';
const app = createApp(App);
app.use(router);
app.use(store);
app.use(ElementUI);
app.mount('#app');
```
#### `src/router/index.js`
```javascript
import { createRouter, createWebHistory } from 'vue-router';
import HomeView from '../views/HomeView.vue';
import LoginView from '../views/LoginView.vue';
import RegisterView from '../views/RegisterView.vue';
import DoctorListView from '../views/DoctorListView.vue';
import AppointmentView from '../views/AppointmentView.vue';
import HistoryView from '../views/HistoryView.vue';
const routes = [
{ path: '/', component: HomeView },
{ path: '/login', component: LoginView },
{ path: '/register', component: RegisterView },
{ path: '/doctors', component: DoctorListView },
{ path: '/appointment', component: AppointmentView },
{ path: '/history', component: HistoryView }
];
const router = createRouter({
history: createWebHistory(),
routes
});
export default router;
```
#### `src/store/index.js`
```javascript
import { createStore } from 'vuex';
export default createStore({
state: {
user: null,
doctors: [],
appointments: []
},
mutations: {
setUser(state, user) {
state.user = user;
},
setDoctors(state, doctors) {
state.doctors = doctors;
},
addAppointment(state, appointment) {
state.appointments.push(appointment);
}
},
actions: {
fetchDoctors({ commit }) {
// 模拟从服务器获取医生列表
const doctors = [
{ id: 1, name: '张三', specialty: '内科', schedule: ['周一上午', '周三下午'] },
{ id: 2, name: '李四', specialty: '外科', schedule: ['周二全天', '周五上午'] }
];
commit('setDoctors', doctors);
}
}
});
```
#### `src/views/HomeView.vue`
```vue
<template>
<div class="home">
<h1>欢迎使用预约挂号系统</h1>
<el-button type="primary" @click="$router.push('/login')">登录</el-button>
<el-button type="success" @click="$router.push('/register')">注册</el-button>
</div>
</template>
<script>
export default {
name: 'HomeView'
};
</script>
<style scoped>
.home {
text-align: center;
margin-top: 50px;
}
</style>
```
#### `src/components/DoctorCard.vue`
```vue
<template>
<div class="doctor-card">
<img :src="require(`@/assets/${doctor.image}`)" alt="医生照片"> <!-- 需要添加医生的照片 -->
<h3>{{ doctor.name }}</h3>
<p>专业: {{ doctor.specialty }}</p>
<p>擅长: {{ doctor.expertise }}</p>
<p>排班: {{ doctor.schedule.join(', ') }}</p>
<el-button type="primary" @click="selectDoctor">选择医生</el-button>
</div>
</template>
<script>
export default {
props: {
doctor: Object
},
methods: {
selectDoctor() {
this.$emit('select', this.doctor);
}
}
};
</script>
<style scoped>
.doctor-card {
border: 1px solid #ccc;
padding: 20px;
margin: 10px;
display: inline-block;
width: 200px;
text-align: center;
}
</style>
```
#### `src/views/DoctorListView.vue`
```vue
<template>
<div class="doctor-list">
<h1>医生列表</h1>
<div v-for="doctor in doctors" :key="doctor.id">
<DoctorCard :doctor="doctor" @select="selectDoctor" />
</div>
</div>
</template>
<script>
import DoctorCard from '@/components/DoctorCard.vue';
export default {
name: 'DoctorListView',
components: {
DoctorCard
},
data() {
return {
doctors: []
};
},
created() {
this.fetchDoctors();
},
methods: {
fetchDoctors() {
this.$store.dispatch('fetchDoctors').then(() => {
this.doctors = this.$store.state.doctors;
});
},
selectDoctor(doctor) {
this.$router.push({ name: 'Appointment', params: { doctorId: doctor.id } });
}
}
};
</script>
<style scoped>
.doctor-list {
text-align: center;
margin-top: 50px;
}
</style>
```
### 其他视图和表单组件
类似地,你可以创建其他视图和表单组件(如 `LoginView.vue`、`RegisterView.vue`、`UserInformationForm.vue` 等),并根据需求实现相应的功能。
### 注意事项
1. **静态资源**:确保在 `src/assets` 目录下添加所需的图片和其他静态资源。
2. **样式调整**:根据实际需求调整样式,确保界面美观且用户体验良好。
3. **API调用**:如果需要从后端获取数据,可以在 `actions` 中实现 API 调用,并处理响应数据。
希望这些代码示例对你有所帮助!如果有任何具体问题或需要进一步的帮助,请随时告知。
阅读全文
相关推荐
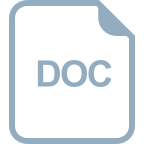
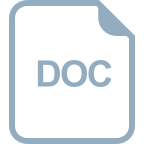
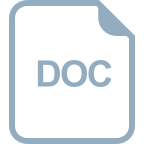
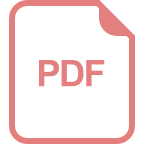
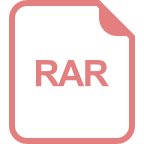
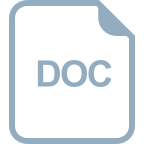
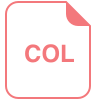
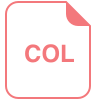
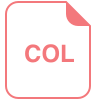
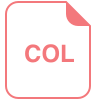
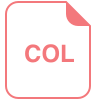
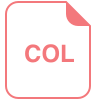
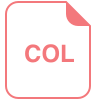
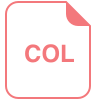
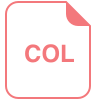
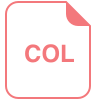
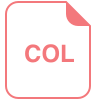
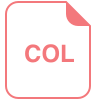
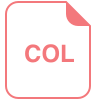