如果我想自己建立KMeans这个函数我该如何建立
时间: 2024-09-16 11:03:41 浏览: 32
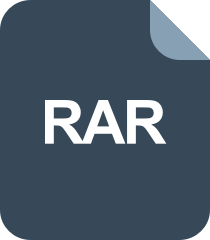
基于matlab实现Copula风光联合场景生成-Kmeans聚类.rar

如果你想从头开始构建一个简单的k均值聚类函数,你可以按照以下几个步骤来设计。这里我们将简化一些细节,因为实际的实现通常会包含更复杂的功能,例如初始化策略、迭代终止条件等。假设数据已经预处理成向量形式:
1. 定义数据结构:
```python
class Point:
def __init__(self, coordinates):
self.coordinates = coordinates
class Cluster:
def __init__(self):
self.centroid = None
self.points = []
```
2. 初始化阶段:
```python
def initialize_clusters(data, k):
clusters = [Cluster() for _ in range(k)]
# 使用随机选择的方式初始化质心
random_indices = np.random.choice(range(len(data)), k)
for i in random_indices:
clusters[i].centroid = Point(data[i])
return clusters
```
3. 聚类更新:
```python
def update_centroids(clusters, data):
for cluster in clusters:
cluster.points = [Point(point) for point in data if is_close_to(cluster.centroid, point)] # 这里需要一个判断接近的函数
if len(cluster.points) > 0:
cluster.centroid = calculate_new_centroid(cluster.points)
else:
# 如果某个簇为空,可以选择新的初始点或其他策略
pass
```
4. 判断停止条件:
```python
def is_converged(clusters):
return all(distance(cluster1.centroid, cluster2.centroid) < tolerance for cluster1, cluster2 in zip(clusters, clusters[1:]))
def calculate_new_centroid(points):
# 计算新质心的平均坐标
return Point(np.mean([point.coordinates for point in points], axis=0))
```
5. 主循环:
```python
def kmeans_clustering(data, k, max_iterations=100, tolerance=1e-6):
clusters = initialize_clusters(data, k)
for _ in range(max_iterations):
if is_converged(clusters):
break
update_centroids(clusters, data)
return clusters
```
注意这只是一个基本版本,实际应用中可能需要添加更多功能如异常处理、距离计算函数等,并考虑如何处理异常值和噪声。同时,上述代码没有涵盖如何计算数据点到质心的距离,这里简单地省略了。
阅读全文
相关推荐
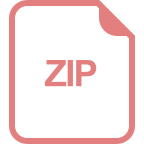
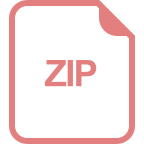
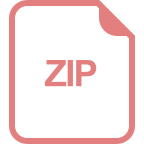
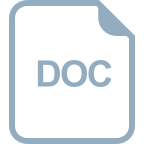
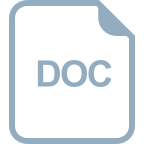
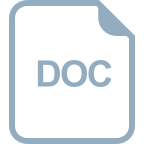
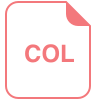
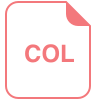
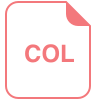
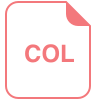
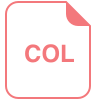






