编写程序:从键盘输入2024级计算机类学生某门课的成绩(学生人数不超过60人),当输入为负时,表示输入结束,试编程①计算并打印最高分②将分数按从高到低顺序进行排序输出 使用c语言解决
时间: 2024-12-17 17:56:43 浏览: 10
在C语言中,你可以使用数组和循环结构来实现这个需求。首先,我们需要创建一个数组存储学生的成绩,然后我们遍历数组,处理用户输入,统计最高分,并保存最高分的索引。同时,我们可以使用选择排序算法对成绩进行降序排列。以下是详细的代码示例:
```c
#include <stdio.h>
#define MAX_STUDENTS 60
void swap(int *a, int *b) {
int temp = *a;
*a = *b;
*b = temp;
}
void selectionSort(int arr[], int n) {
for (int i = 0; i < n - 1; i++) {
int max_idx = i;
for (int j = i + 1; j < n; j++) {
if (arr[j] > arr[max_idx]) {
max_idx = j;
}
}
swap(&arr[i], &arr[max_idx]);
}
}
int main() {
int scores[MAX_STUDENTS];
int studentCount = 0;
while (studentCount < MAX_STUDENTS) {
int score;
printf("请输入2024级计算机类学生某门课的成绩(输入负数结束): ");
scanf("%d", &score);
if (score < 0) break;
scores[studentCount++] = score;
}
if (studentCount > 0) {
int highestScore = scores[0];
printf("最高分为: %d\n", highestScore);
selectionSort(scores, studentCount);
printf("成绩按照从高到低排序:\n");
for (int i = 0; i < studentCount; i++) {
printf("%d ", scores[i]);
}
} else {
printf("没有输入任何成绩。\n");
}
return 0;
}
```
这段代码首先初始化一个数组`scores`,然后读取用户的输入直到遇到负数。接着,如果输入了成绩,则计算最高分并将其保存。最后,使用选择排序对成绩数组进行降序排序并打印结果。
阅读全文
相关推荐
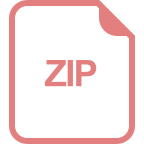
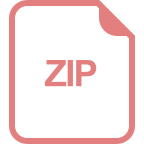
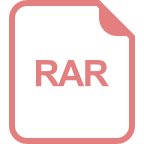


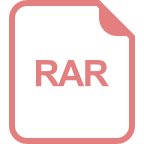
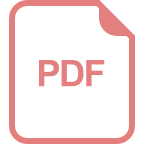
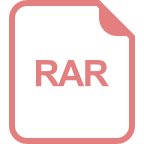
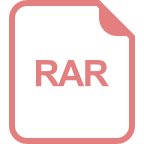
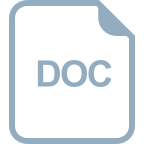
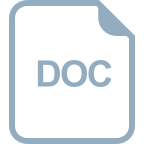
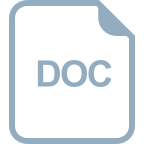
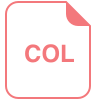




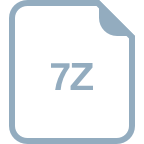
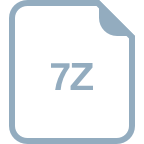
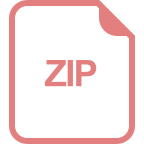