优化这段代码:typedef struct node * PNode; typedef struct node { Frame frame; PNode next; }Node;
时间: 2024-03-12 07:11:23 浏览: 106
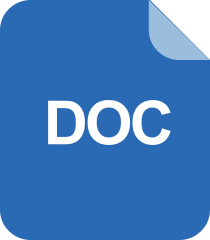
C语言中typedef用法-综合文档
可以将结构体定义与指针别名的声明合并在一起,优化后的代码如下:
```c
typedef struct node
{
Frame frame;
struct node* next;
} Node, *PNode;
```
这样定义结构体时可以直接使用 `Node` 和 `PNode`,不需要再分别声明结构体和指针别名。
阅读全文
相关推荐
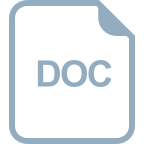
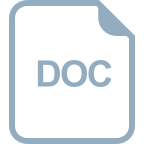



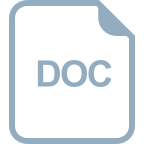




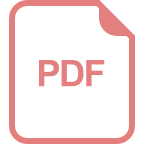
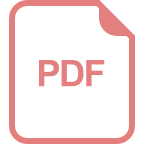





