C#画一个简单的爱心动画
时间: 2024-09-06 18:05:00 浏览: 89
在C#中,我们可以使用Windows Forms或WPF等图形库来创建一个简单的爱心动画。这里我将以Windows Forms为例,因为它的API相对直观。首先,你需要创建一个新的Windows Form应用程序,并添加一个PictureBox控件作为绘制区域。
1. **步骤1**: 创建爱心形状**
使用Graphics类的DrawPolygon方法,画出一个心形。这需要两个点对(上半部分和下半部分),每个点对由三个点组成,形成类似"Π"的形状。
```csharp
private void DrawHeart(Graphics g, Color color)
{
int[] xPoints = { 0, 75, 136, 75, 0 };
int[] yPoints = { 0, 0, 80, 150, 0 };
// 绘制爱心
g.FillPolygon(color, new Point[xPoints.Length], new int[] { xPoints, yPoints });
}
```
2. **步骤2**: 动画效果**
我们可以使用Timer控件定时更新PictureBox的内容。当`Tick`事件触发时,我们改变绘制的心形位置或旋转角度,模拟动画效果。
```csharp
private Timer animationTimer;
private int angle = 0;
private void StartAnimation()
{
animationTimer = new Timer();
animationTimer.Interval = 10; // 每隔10毫秒执行一次
animationTimer.Tick += AnimationTick;
animationTimer.Start();
}
private void AnimationTick(object sender, EventArgs e)
{
// 更新角度并重新绘制
angle += 5; // 可调整步长
if (angle > 360) angle = 0;
Invalidate(); // 刷新PictureBox
// 添加更复杂的动画效果,如平移、缩放或颜色变化
// ...
}
```
3. **步骤3**: 调用函数并开始动画**
在Form的Load事件中初始化并启动动画。
```csharp
protected override void OnLoad(EventArgs e)
{
base.OnLoad(e);
DrawHeart(PictureBox1.CreateGraphics(), Color.Red); // 初始化显示红色爱心
StartAnimation();
}
```
4. **相关问题**:
1. 如何让爱心在多个位置间移动而非仅旋转?
2. 如何在WPF中实现类似的效果?
3. 如何控制动画的速度?
阅读全文
相关推荐
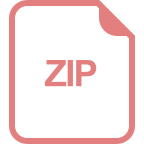
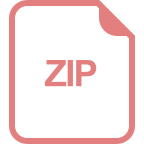
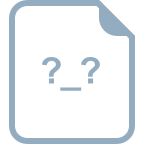
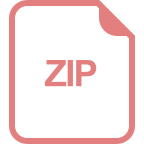
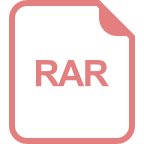
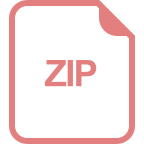
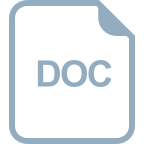
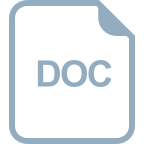
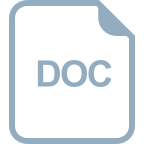
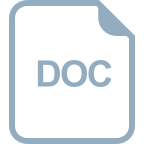







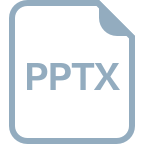
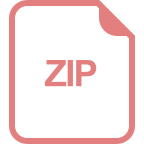