c++11 copy_file_range 使用方法
时间: 2024-09-06 21:01:32 浏览: 22
`copy_file_range`是自C++11标准引入的,它允许高效地复制文件的部分内容,尤其是对于大型文件的局部更新非常有用。这个功能位于`<sys/sendfile.h>`头文件中,但在某些旧的系统或库可能不支持。以下是`copy_file_range`的基本用法:
```cpp
#include <sys/sendfile.h>
#include <unistd.h> // 包含SEEK_SET常量
// 参数说明:
// out_fd: 输出文件描述符(通常是打开的目标文件)
// in_fd: 输入文件描述符(通常是打开的源文件)
// out_offset: 输出文件中的偏移位置
// in_offset: 输入文件中的偏移位置
// count: 要复制的字节数
size_t copy_file_range(int out_fd, int in_fd, off64_t out_offset, off64_t in_offset, size_t count) {
if (copy_file_range(out_fd, in_fd, static_cast<off_t>(out_offset), static_cast<off_t>(in_offset), count) != count) {
perror("Error copying file range");
return -1; // 返回错误状态
}
return count;
}
// 示例:
int src_fd = open("sourcefile", O_RDONLY);
int dst_fd = open("destinationfile", O_WRONLY);
// 检查是否成功打开文件
if (src_fd < 0 || dst_fd < 0) {
// 处理错误
}
// 设置输入和输出偏移开始复制部分
off64_t start_in = ...; // 从sourcefile的某个位置开始
off64_t start_out = ...; // 从destinationfile的某个位置开始
// 复制文件的一部分
size_t copied_bytes = copy_file_range(dst_fd, src_fd, start_out, start_in, sizeof(buffer));
close(src_fd);
close(dst_fd);
// 如果复制出错,这里会捕获到错误信息并处理
```
在这个例子中,你需要先确定好`start_in`和`start_out`的位置,然后调用`copy_file_range`进行复制。这个函数内部会进行高效的直接数据块传输,减少了内存开销。
注意,`off64_t`用于跨平台处理大文件的偏移量,如果只涉及小文件,可以使用`off_t`代替。
相关推荐
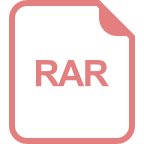
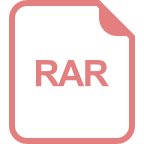















