C++ cout __func__
时间: 2023-10-22 10:06:52 浏览: 106
`__func__` 是一个预定义的标识符,表示当前函数或方法的名称。在 C++ 中,您可以使用 `cout` 来输出 `__func__`,以便在程序运行时查看当前函数或方法的名称。例如:
```c++
#include <iostream>
void myFunction() {
std::cout << "Current function: " << __func__ << std::endl;
}
int main() {
myFunction();
return 0;
}
```
输出将是:
```
Current function: myFunction
```
相关问题
c++ _func_ _LINE_
C++ 中的 _func_ 和 _LINE_ 是预定义宏,用于调试时输出当前函数名和代码行数。它们可以在程序中直接使用,不需要进行定义。
具体来说,_func_ 用于输出当前函数名,例如:
```
cout << "当前所在函数名为:" << __func__ << endl;
```
而 _LINE_ 则用于输出当前代码行数,例如:
```
cout << "当前代码所在行数为:" << __LINE__ << endl;
```
这两个预定义宏在调试时非常有用,可以方便地定位出错的位置。同时也可以帮助开发人员更好地了解程序的执行过程。
C++ pthread_create demo
下面是一个使用 `pthread_create` 创建线程的 C++ 示例代码:
```c++
#include <iostream>
#include <pthread.h>
using namespace std;
void* thread_func(void* arg)
{
int* p = (int*)arg;
cout << "Thread ID: " << pthread_self() << ", Arg: " << *p << endl;
return NULL;
}
int main()
{
pthread_t tid;
int arg = 12345;
// 创建线程
if (pthread_create(&tid, NULL, thread_func, &arg) != 0) {
cout << "Error: Failed to create thread." << endl;
return -1;
}
// 等待线程结束
if (pthread_join(tid, NULL) != 0) {
cout << "Error: Failed to join thread." << endl;
return -1;
}
cout << "Main thread exit." << endl;
return 0;
}
```
在上面的示例代码中,我们首先定义了一个名为 `thread_func` 的函数,这个函数将作为线程的入口函数。在 `thread_func` 函数中,我们打印了当前线程的 ID 和传入的参数值,然后返回 NULL。
在主函数中,我们首先定义了一个 `pthread_t` 类型的变量 `tid`,用于存储线程 ID。然后定义了一个整型变量 `arg`,用于向线程传递参数。接着,我们使用 `pthread_create` 函数创建了一个新线程,并将线程 ID 存储在 `tid` 变量中。在调用 `pthread_create` 函数时,我们传入了四个参数:
- 第一个参数是指向线程 ID 变量的指针。
- 第二个参数是线程属性,我们将其设置为 NULL。
- 第三个参数是指向线程入口函数的指针,即 `thread_func` 函数。
- 第四个参数是传递给线程入口函数的参数,即 `arg` 变量的地址。
接下来,我们使用 `pthread_join` 函数等待线程结束。在调用 `pthread_join` 函数时,我们传入了两个参数:
- 第一个参数是要等待的线程 ID。
- 第二个参数是指向线程返回值的指针,我们将其设置为 NULL,表示不关心线程的返回值。
最后,我们在主函数中打印了一条消息,表示主线程退出。
阅读全文
相关推荐
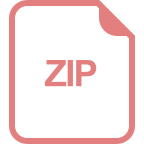
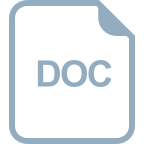
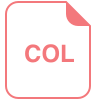
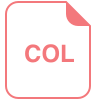
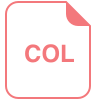



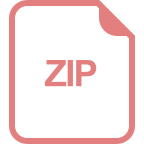
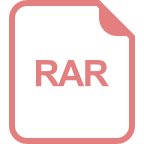
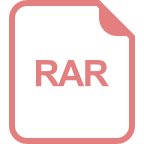


