python实现英雄飞机和敌机的碰撞检测,并触发销毁
时间: 2024-11-06 10:16:23 浏览: 7
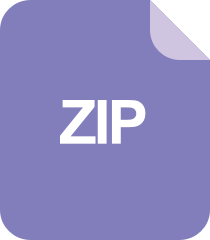
python基于percols算法的疲劳检测

在Python中,为了实现英雄飞机和敌机的碰撞检测并触发销毁,你可以使用游戏开发库如Pygame,它提供了一套处理像素级位置和碰撞的基本工具。这里是一个简单的示例:
首先,你需要定义一个类来表示飞机,包括它的位置、尺寸以及是否存活的状态。例如:
```python
import pygame
class Plane(pygame.sprite.Sprite):
def __init__(self, pos, image_path, size=(50, 50)):
super().__init__()
self.image = pygame.image.load(image_path)
self.rect = self.image.get_rect(center=pos)
self.size = size
self.is_alive = True
def collide(self, other):
return self.rect.colliderect(other.rect)
```
然后,在游戏循环中,对所有英雄飞机和敌机实例执行碰撞检测:
```python
def check_collision(player_planes, enemy_planes):
for player in player_planes:
for enemy in enemy_planes:
if player.collide(enemy):
player.is_alive = False
print(f"Player plane collided with enemy at {player.rect.center}")
```
最后,在更新屏幕前检查每个飞机的存活状态并作出相应的反应:
```python
for plane in player_planes + enemy_planes:
if not plane.is_alive:
plane.kill() # 使用pygame的kill方法从屏幕上移除已销毁的飞机
```
阅读全文
相关推荐
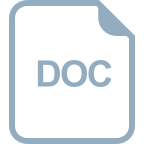
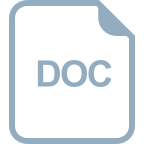


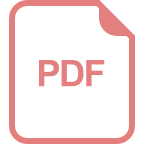
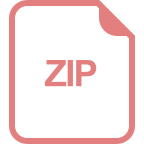
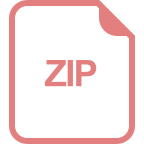
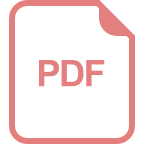
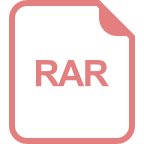
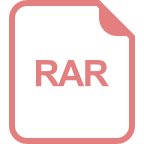
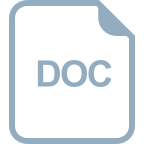
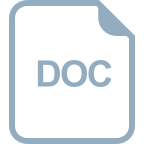
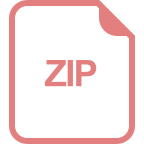
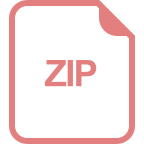
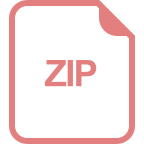