python巴特沃斯低通和高通滤波器
时间: 2023-10-29 08:06:48 浏览: 57
巴特沃斯滤波器是一种常用的数字滤波器,可以用于信号处理中的低通滤波和高通滤波。它的特点是在通带内具有平坦的幅频响应,而在截止频率附近具有较为陡峭的衰减特性。
Python中可以使用scipy库中的signal模块来实现巴特沃斯滤波器。具体实现步骤如下:
1. 导入需要的库
```python
from scipy import signal
import matplotlib.pyplot as plt
import numpy as np
```
2. 定义滤波器参数
```python
# 采样频率
fs = 1000.0
# 截止频率
fc = 50.0
# 通带最大衰减
rp = 1.0
# 阻带最小衰减
rs = 60.0
# 滤波器类型(低通或高通)
ftype = 'lowpass'
```
3. 计算滤波器系数
```python
# 计算阶数和截止频率
order, Wn = signal.buttord(2 * fc / fs, 2 * (fc + 10) / fs, rp, rs)
# 计算滤波器系数
b, a = signal.butter(order, Wn, btype=ftype)
```
4. 使用滤波器进行信号处理
```python
# 生成测试信号
t = np.linspace(0, 1, 1000, endpoint=False)
sig = np.sin(2 * np.pi * 50 * t) + 0.5 * np.sin(2 * np.pi * 200 * t)
使用滤波器进行信号处理
filtered_sig = signal.filtfilt(b, a, sig)
```
5. 绘制滤波前后的信号图像
```python
# 绘制滤波前后的信号图像
fig, (ax1, ax2) = plt.subplots(2, 1, sharex=True)
ax1.plot(t, sig)
ax1.set_title('Original signal')
ax1.axis([0, 1, -2, 2])
ax2.plot(t, filtered_sig)
ax2.set_title('Filtered signal')
ax2.axis([0, 1, -2, 2])
plt.tight_layout()
plt.show()
```
巴特沃斯高通滤波器的实现与低通滤波器类似,只需要将滤波器类型改为'highpass'即可。
相关推荐
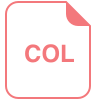
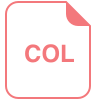
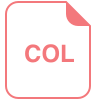
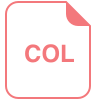
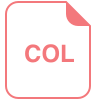









