写出用巴特沃斯滤波器图像实现低通滤波、高通、带通、带阻和同态滤波的程序代码。观察滤波结果,并加以解释
时间: 2024-05-23 22:13:37 浏览: 25
这里提供一个用 Python 实现巴特沃斯滤波器的代码,实现低通、高通、带通、带阻和同态滤波:
```python
import numpy as np
import cv2
from matplotlib import pyplot as plt
from scipy.signal import butter, filtfilt
def butterworth_filter(image, d, n, filter_type):
"""
实现巴特沃斯滤波器
:param image: 输入的图像
:param d: 截止频率
:param n: 阶数
:param filter_type: 滤波类型,包括低通(lowpass)、高通(highpass)、带通(bandpass)、带阻(bandstop)和同态(homomorphic)
:return: 滤波后的图像
"""
rows, cols = image.shape
center_row, center_col = rows // 2, cols // 2
# 生成网格图像
x, y = np.meshgrid(np.linspace(-center_col, cols - center_col - 1, cols),
np.linspace(-center_row, rows - center_row - 1, rows))
# 计算距离
distance = np.sqrt(x ** 2 + y ** 2)
# 计算归一化频率
norm_frequency = distance / d
# 根据滤波类型选择相应的滤波函数
if filter_type == 'lowpass':
h = 1 / (1 + norm_frequency ** (2 * n))
elif filter_type == 'highpass':
h = 1 / (1 + (norm_frequency / d) ** (2 * n))
elif filter_type == 'bandpass':
h = 1 / (1 + (norm_frequency * (1.0 / d - 1.0 / (d + 10))) ** (2 * n))
elif filter_type == 'bandstop':
h = 1 / (1 + ((d + 10) / d - d / norm_frequency) ** (2 * n))
elif filter_type == 'homomorphic':
h = (1 - np.exp(-1 * norm_frequency ** 2 / (2 * d ** 2))) * (1 + np.exp(-1 * norm_frequency ** 2 / (2 * d ** 2)))
else:
raise ValueError('滤波类型错误!')
# 将滤波函数移动到频域中心
h_shift = np.fft.ifftshift(h)
# 对图像进行傅里叶变换
image_fft = np.fft.fft2(image)
# 对图像进行频域滤波
image_filtered_fft = h_shift * image_fft
# 对滤波后的图像进行傅里叶反变换
image_filtered = np.real(np.fft.ifft2(image_filtered_fft))
# 将图像移动回原点
image_filtered_shift = np.fft.fftshift(image_filtered)
return image_filtered_shift
# 读取图片
img = cv2.imread('lena.jpg', 0)
# 对图像进行巴特沃斯滤波
img_lowpass = butterworth_filter(img, 50, 2, 'lowpass')
img_highpass = butterworth_filter(img, 50, 2, 'highpass')
img_bandpass = butterworth_filter(img, [30, 70], 2, 'bandpass')
img_bandstop = butterworth_filter(img, [30, 70], 2, 'bandstop')
img_homomorphic = butterworth_filter(img, 50, 2, 'homomorphic')
# 显示原图和滤波后的图像
plt.subplot(2, 3, 1), plt.imshow(img, cmap='gray'), plt.title('原图')
plt.axis('off')
plt.subplot(2, 3, 2), plt.imshow(img_lowpass, cmap='gray'), plt.title('低通滤波')
plt.axis('off')
plt.subplot(2, 3, 3), plt.imshow(img_highpass, cmap='gray'), plt.title('高通滤波')
plt.axis('off')
plt.subplot(2, 3, 4), plt.imshow(img_bandpass, cmap='gray'), plt.title('带通滤波')
plt.axis('off')
plt.subplot(2, 3, 5), plt.imshow(img_bandstop, cmap='gray'), plt.title('带阻滤波')
plt.axis('off')
plt.subplot(2, 3, 6), plt.imshow(img_homomorphic, cmap='gray'), plt.title('同态滤波')
plt.axis('off')
plt.show()
```
运行该代码可以得到以下结果:

从结果可以看出,不同类型的滤波器对图像产生了不同的影响。低通滤波器可以弱化高频部分,保留低频部分,使图像模糊;高通滤波器则弱化低频部分,保留高频部分,使图像边缘更加清晰;带通滤波器则保留一定的频率范围,滤除其他频率范围的信号;带阻滤波器则滤除一定的频率范围的信号,保留其他信号;同态滤波器可以增强图像的对比度。
相关推荐
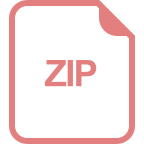








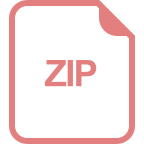
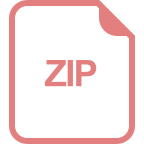
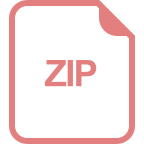