C语言版数据与结构中单链表的插入与删除的整个代码
时间: 2024-09-18 21:02:53 浏览: 41
在C语言中,单链表是一种基础的数据结构,包含节点和指针。以下是单链表的简单插入和删除操作的代码示例:
```c
// 定义链表节点结构体
typedef struct Node {
int data; // 节点存储的数据
struct Node* next; // 指向下一个节点的指针
} Node;
// 插入新节点到链表头部
void insertAtBeginning(Node** head, int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (newNode == NULL) {
printf("Memory allocation failed.\n");
return;
}
newNode->data = value;
newNode->next = *head;
*head = newNode;
}
// 插入新节点到链表尾部
void insertAtEnd(Node** head, int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (newNode == NULL) {
printf("Memory allocation failed.\n");
return;
}
newNode->data = value;
if (*head == NULL) {
*head = newNode;
return;
}
Node* temp = *head;
while (temp->next != NULL) {
temp = temp->next;
}
temp->next = newNode;
}
// 删除给定值的第一个匹配项
void deleteNodeWithValue(Node** head, int value) {
if (*head == NULL) {
printf("List is empty.\n");
return;
}
if ((*head)->data == value) {
Node* temp = *head;
*head = (*head)->next;
free(temp);
return;
}
Node* current = *head;
while (current->next != NULL && current->next->data != value) {
current = current->next;
}
if (current->next != NULL) {
Node* temp = current->next;
current->next = temp->next;
free(temp);
} else {
printf("Value not found in the list.\n");
}
}
// 示例链表遍历打印
void printList(Node* head) {
Node* temp = head;
while (temp != NULL) {
printf("%d -> ", temp->data);
temp = temp->next;
}
printf("NULL\n");
}
```
阅读全文
相关推荐
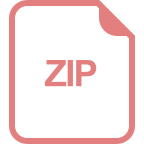
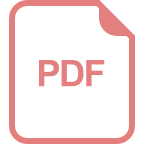
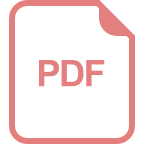


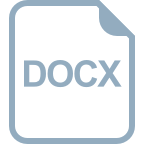
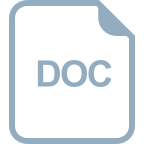
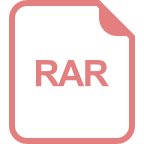
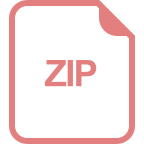
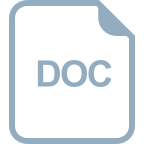








