python 乘除法
时间: 2023-10-28 17:57:56 浏览: 82
Python中的乘法和除法运算符分别是*和/。你可以使用*来进行乘法运算,例如:
```python
x = 5 * 3
print(x) # 输出结果为 15
```
同样地,你可以使用/来进行除法运算,例如:
```python
y = 10 / 2
print(y) # 输出结果为 5.0
```
需要注意的是,使用/进行除法运算时,结果会自动转换为浮点数。如果你想要得到整数结果,可以使用//运算符,例如:
```python
z = 10 // 3
print(z) # 输出结果为 3
```
这样可以得到整数部分的结果。如果你只想得到除法的余数,可以使用%运算符,例如:
```python
remainder = 10 % 3
print(remainder) # 输出结果为 1
```
这样会得到除法的余数部分。希望这能解答你的问题!如果还有其他问题,请继续提问。
相关问题
生成pathon乘除法表代码
当然可以,以下是一个简单的示例代码:
```python
for i in range(1, 10):
for j in range(1, 10):
print("{0}*{1}={2}\t".format(i, j, i*j), end='')
print("")
```
希望这个回答能够帮助到您!
python将csv文件里的字符串转化为数值再进行乘除法计算的代码
好的,以下是Python将CSV文件里的字符串转化为数值再进行乘除法计算的代码:
```
import csv
with open('file.csv') as file:
reader = csv.reader(file)
next(reader) # 跳过表头
for row in reader:
value1 = float(row[0])
value2 = float(row[1])
result = value1 * value2 / 100
print('Result: ', result)
```
这段代码可以将“file.csv”文件中的数据读取出来,将第一列数据转换为浮点型的数值“value1”,第二列数据转换为浮点型的数值“value2”,然后进行乘除法计算,并输出结果。如果“file.csv”文件的格式不是标准的CSV格式,或者文件中包含非数值的字符,那么程序可能会出现错误。
相关推荐
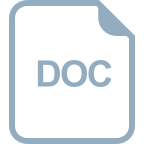
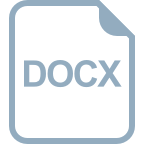












