python音乐播放助手代码(带界面音乐歌手序号播放暂停)
时间: 2024-10-28 19:12:38 浏览: 20
在Python中,可以使用`tkinter`库结合`pyglet`或`pygame`来创建一个基本的音乐播放助手,它们都支持播放音频并提供交互式界面。这里给出一个使用`pyglet`的例子,它有基本的播放、暂停以及显示歌曲信息的功能:
```python
import pyglet
from tkinter import Tk, Label, Button
class MusicPlayer:
def __init__(self, music_file):
self.music = pyglet.media.load(music_file)
self.window = Tk()
self.current_song = 0
self.label = Label(text=f"歌曲{self.current_song + 1}: 歌手未知")
self.play_button = Button(text="播放", command=self.play_music)
self.pause_button = Button(text="暂停", command=self.pause_music)
def play_music(self):
self.player = pyglet.media.Player()
self.player.queue(self.music)
self.player.play()
self.window.title(f"歌曲{self.current_song + 1}")
self.label.config(text=f"歌曲{self.current_song + 1}: 歌手未知")
def pause_music(self):
self.player.pause()
def stop_music(self):
self.player.stop()
self.window.title("已停止")
def on_playback_finish(self):
self.current_song += 1
if self.current_song < len(music_files): # 假设music_files是包含所有歌曲的列表
next_song = pyglet.media.load(music_files[self.current_song])
self.player.queue(next_song)
self.player.play()
self.label.config(text=f"歌曲{self.current_song + 1}: 歌手未知")
else:
self.stop_music()
player = MusicPlayer('your_music.mp3') # 替换为你的音乐文件
player.window.mainloop()
```
请注意,这只是一个基础版示例,实际应用可能需要处理更多的细节,例如加载更多歌曲、添加进度条等。
阅读全文
相关推荐
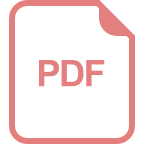
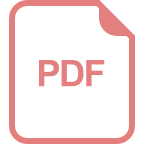
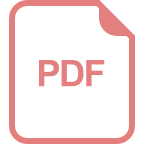
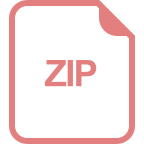
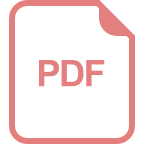
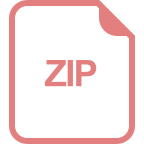
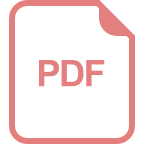
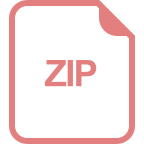
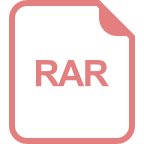
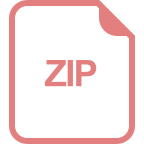
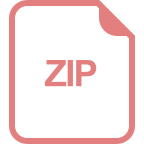
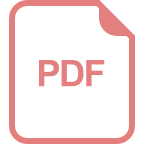
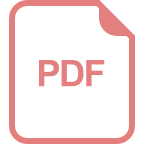
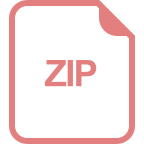