springboot接收数据流
时间: 2023-10-17 15:34:08 浏览: 64
在Spring Boot中接收数据流可以通过以下步骤进行操作:
1. 在你的控制器方法中,使用`@RequestBody`注解来接收数据流。例如:
```java
@PostMapping("/upload")
public void uploadFile(@RequestBody InputStream fileStream) {
// 处理文件流
// ...
}
```
2. 确保你的请求头中包含正确的`Content-Type`,例如`Content-Type: application/octet-stream`。
3. 在请求体中发送数据流。你可以使用一些工具,例如curl或Postman,来发送包含数据流的POST请求。
这样,当你发送带有数据流的POST请求到`/upload`路径时,Spring Boot将会将请求体中的数据流注入到`fileStream`参数中,你可以在方法中对其进行处理。
请确保你已经添加了Spring Boot的相关依赖,例如`spring-boot-starter-web`,以便使用注解和其他相关功能。
相关问题
SpringBoot接收参数实验心得
SpringBoot是一个非常流行的Java开发框架,它提供了很多方便的功能来简化开发过程。在SpringBoot中,我们可以使用@Controller注解来定义控制器,使用@RequestMapping注解来定义请求路由。
当我们需要接收参数时,可以使用@RequestParam注解来将请求参数绑定到方法参数上。例如:
```java
@Controller
public class UserController {
@RequestMapping("/user")
public String getUser(@RequestParam("id") int id, Model model) {
// 根据id查询用户信息
User user = userService.getUserById(id);
// 将用户信息添加到Model中
model.addAttribute("user", user);
// 返回用户详情页面
return "userDetail";
}
}
```
上面的代码中,我们使用@RequestParam注解将请求参数id绑定到方法参数id上。当请求/user?id=1时,SpringBoot会自动将id的值设置为1,并调用getUser方法。
除了@RequestParam注解外,还有@PathVariable注解可以用来获取路径变量,@RequestBody注解可以用来获取请求体中的数据。
在实际开发中,我们需要注意参数的类型和名称,以及参数是否必须等问题。同时,为了保证接口的安全性,我们还需要对参数进行校验和过滤,避免恶意攻击和非法操作。
java springboot接收InputStream参数
在Spring Boot中,可以使用`@RequestBody`注解来接收InputStream类型的参数。下面是一个示例:
```java
@PostMapping("/upload")
public String uploadFile(@RequestBody InputStream inputStream) {
// 处理文件上传逻辑
return "file uploaded successfully";
}
```
在上面的示例中,我们使用`@RequestBody`注解将InputStream类型的参数注入到方法中,并处理文件上传逻辑。当客户端发送POST请求时,将请求体中的数据读取为InputStream类型,并传递给该方法进行处理。
需要注意的是,由于InputStream是一个流,它只能读取一次。如果需要多次读取该InputStream,需要进行缓存。另外,需要确保在处理完该InputStream后,关闭流以释放资源。
相关推荐
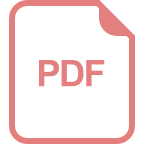












