在C++中,如何将字符串'boot'转换为整数类型(int)?
时间: 2024-10-22 14:08:20 浏览: 13
在C++中,如果你想将字符串'boot'(这里假设它是一个可以解析为数字的字符串)转换为整数类型(int),你可以使用`std::stoi`函数。但是,如果'boot'不是一个有效的数字字符串,这个操作会抛出异常。这是一个示例:
```cpp
#include <iostream>
#include <string>
int main() {
std::string str = "boot"; // 假设这是你要转换的字符串
try {
int num = std::stoi(str);
std::cout << "转换后的整数是: " << num << std::endl;
} catch (const std::invalid_argument& e) {
std::cerr << "无法转换为整数: " << e.what() << std::endl;
} catch (const std::out_of_range& e) {
std::cerr << "数值超出范围: " << e.what() << std::endl;
}
return 0;
}
```
在这个例子中,如果'boot'能成功转换成整数,`std::stoi`会返回该整数;如果不能,则会捕获并处理相应的异常。
相关问题
android::base::boot_clock类型怎么转换为chrono::system_clock类型的时间,然后继续打印
要将`android::base::boot_clock`类型的时间转换为`chrono::system_clock`类型的时间,可以使用`android::base::boot_clock::to_time_point()`函数将其转换为`std::chrono::system_clock::time_point`类型的时间。然后可以使用`std::chrono::system_clock::to_time_t()`函数将其转换为时间戳或者使用`std::put_time()`函数将其转换为字符串输出。下面是一段示例代码:
```c++
#include <android-base/chrono_utils.h>
#include <chrono>
#include <ctime>
#include <iomanip>
#include <iostream>
int main() {
// 获取当前时间
auto boot_time = android::base::boot_clock::now();
// 转换为system_clock类型的时间
auto system_time = std::chrono::system_clock::from_time_t(
std::chrono::system_clock::to_time_t(
std::chrono::time_point_cast<std::chrono::system_clock::duration>(boot_time)));
// 输出时间戳
auto timestamp = std::chrono::system_clock::to_time_t(system_time);
std::cout << "Timestamp: " << timestamp << std::endl;
// 输出字符串
std::time_t time_t = std::chrono::system_clock::to_time_t(system_time);
std::cout << "String time: " << std::put_time(std::localtime(&time_t), "%Y-%m-%d %H:%M:%S") << std::endl;
return 0;
}
```
在上述代码中,我们使用`android::base::boot_clock::now()`函数获取当前时间,然后使用`std::chrono::time_point_cast<std::chrono::system_clock::duration>()`函数将其转换为`std::chrono::system_clock::time_point`类型的时间。接着,我们可以使用`std::chrono::system_clock::to_time_t()`函数将其转换为时间戳,并输出;或者使用`std::put_time()`函数将其转换为字符串,并输出。请注意,需要包含`<chrono>`和`<ctime>`头文件才能使用`std::chrono`和`std::put_time()`函数。
android::base::boot_clock::time_point类型的时间怎么打印,写一段可以编译运行成功的代码
要打印`android::base::boot_clock::time_point`类型的时间,可以使用`android::base::boot_clock::now()`函数获取当前时间,然后转换为时间戳或字符串输出。下面是一段可以编译运行的C++代码示例:
```c++
#include <android-base/chrono_utils.h>
#include <iostream>
int main() {
// 获取当前时间
auto now = android::base::boot_clock::now();
// 转换为时间戳
auto timestamp = android::base::boot_clock::to_time_t(now);
std::cout << "Timestamp: " << timestamp << std::endl;
// 转换为字符串
auto str_time = android::base::boot_clock::to_string(now);
std::cout << "String time: " << str_time << std::endl;
return 0;
}
```
上述代码中,我们使用`android::base::boot_clock::now()`函数获取当前时间,然后使用`android::base::boot_clock::to_time_t()`函数将时间转换为时间戳,并输出。另外,我们还可以使用`android::base::boot_clock::to_string()`函数将时间转换为字符串,并输出。
阅读全文
相关推荐
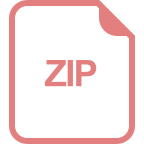
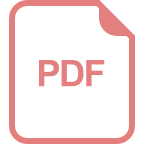
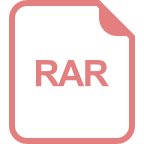
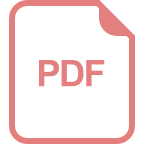
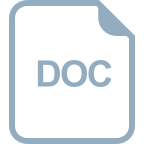
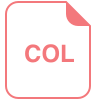
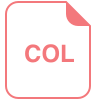
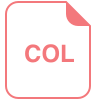
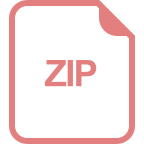