如何在C++中进行加密
时间: 2024-09-27 12:06:18 浏览: 29
在C++中进行加密通常涉及到使用各种密码学算法和库,例如标准库中的`<openssl>`或者第三方库如`Crypto++`、`OpenSSL for Windows`等。这里我会介绍一种基本的方法,使用简单的凯撒密码(Caesar Cipher)作为示例,因为其易于理解但不够安全。
**凯撒密码示例**:
凯撒密码是最简单的替换式加密算法,它将明文中的每个字母按照固定的偏移量移动到字母表的另一个位置。例如,偏移量为3,'A'会变成'D','B'会变成'E',依此类推。
```cpp
#include <iostream>
#include <string>
// 加密函数
std::string caesar_encrypt(const std::string& plaintext, int shift) {
std::string ciphertext;
for (char c : plaintext) {
if (isalpha(c)) { // 检查字符是否为字母
if (isupper(c)) {
ciphertext += static_cast<char>((c - 'A' + shift) % 26 + 'A');
} else {
ciphertext += static_cast<char>((c - 'a' + shift) % 26 + 'a');
}
} else {
ciphertext += c; // 非字母字符保持不变
}
}
return ciphertext;
}
// 示例用法
int main() {
std::string plain_text = "Hello, World!";
int shift = 3;
std::string encrypted_text = caesar_encrypt(plain_text, shift);
std::cout << "Original text: " << plain_text << "\n";
std::cout << "Encrypted text: " << encrypted_text << "\n";
return 0;
}
```
阅读全文
相关推荐
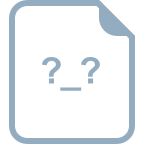
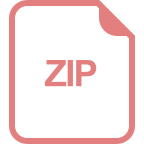
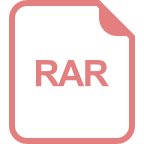
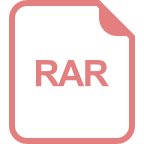
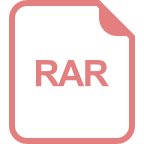
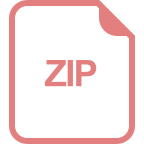
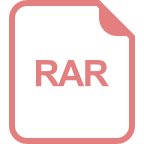
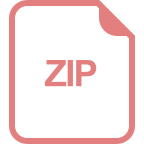
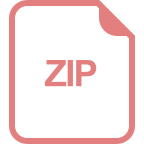
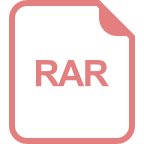
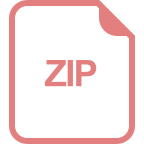
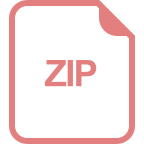
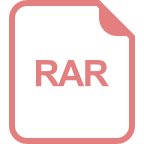