python函数差分处理
时间: 2024-06-16 11:01:03 浏览: 18
在Python中,函数差分处理通常是指计算两个连续函数值之间的差异,特别是在信号处理、数据分析或数学建模中,这可以用来估计数据的斜率、变化率或瞬时速度。Python提供了多种库来进行这个操作,比如NumPy和SciPy。
**1. NumPy中的差分**:
NumPy的`numpy.diff()`函数可以用来计算数组元素之间的差分。例如:
```python
import numpy as np
arr = np.array([1, 2, 3, 4, 5])
diff = np.diff(arr)
```
这里`diff`将是一个一维数组,表示原数组的逐元素差分:[1, 1, 1].
**2. SciPy中的差分**:
SciPy的`scipy.signal`模块提供了更高级的差分和滤波工具。例如,`scipy.signal.slow_filter()`函数可以进行滑动窗口差分:
```python
from scipy.signal import savgol_filter
windowed_diff = savgol_filter(arr, 51, 2) # 51是窗口大小,2是polyfit次数
```
**3. 自定义差分**:
如果需要更复杂的自定义差分规则(如中心差分、二阶导数等),可以编写循环或者利用NumPy的向量化操作,结合条件索引等。
**相关问题**:
1. NumPy的`diff()`函数默认使用什么方法计算差分?
2. SciPy的`savgol_filter()`函数主要用于哪种类型的差分?
3. 如何在Python中实现二阶导数的计算?
相关问题
python二阶差分
Python的二阶差分是指对一个数组进行两次差分操作,即先对数组中的元素依次做一次一阶差分,再对计算得到的一阶差分结果再做一次一阶差分。
实现二阶差分的方法可以利用Python中的numpy库和diff()函数来实现。首先,导入numpy库:
```
import numpy as np
```
接下来,定义一个数组x,我们以[1, 3, 7, 10, 6]为例:
```
x = np.array([1, 3, 7, 10, 6])
```
然后,利用numpy的diff()函数进行一阶差分操作:
```
diff1 = np.diff(x)
```
一阶差分操作得到的结果是[2, 4, 3, -4]。再对结果diff1再次进行一阶差分操作:
```
diff2 = np.diff(diff1)
```
二阶差分操作得到的结果是[2, -1, -7]。即对原始数组x进行两次差分操作,最终得到了二阶差分的结果。
二阶差分在数据分析和时间序列分析中经常用于数据处理和特征提取。它可以帮助我们查找数据的趋势和周期性变化,同时也可以用于异常检测和去除数据中的噪声。通过Python中的numpy库,实现二阶差分非常简便。
python三重差分
三重差分法是双重差分法的进一步改进,用于处理控制组和实验组的时间趋势不同的情况。下面是一个使用Python进行三重差分的例子:
假设我们有一个数据集,其中包含了控制组和实验组的数据,以及时间和其他一些控制变量。我们想要估计政策对企业生产效率的影响,但是我们发现控制组和实验组的时间趋势不同。我们可以使用三重差分法来解决这个问题。
首先,我们需要对数据进行预处理,将数据按照时间和组别进行排序。然后,我们可以使用pandas库来计算三重差分估计量。下面是一个示例代码:
```python
import pandas as pd
import statsmodels.formula.api as smf
# 读取数据
data = pd.read_csv('data.csv')
# 对数据按照时间和组别进行排序
data = data.sort_values(['time', 'group'])
# 计算三重差分估计量
model = smf.ols('y ~ treatment + time + group + treatment:time + treatment:group + time:group + treatment:time:group', data=data)
result = model.fit()
# 输出结果
print(result.summary())
```
在上面的代码中,我们使用了statsmodels库来进行回归分析。我们首先将数据按照时间和组别进行排序,然后使用ols函数来拟合一个多元线性回归模型。在模型中,我们包含了treatment、time、group以及它们的交互项,这样就可以计算出三重差分估计量。最后,我们使用summary函数来输出回归结果。
相关推荐
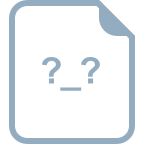
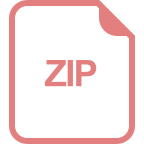












