RSA密码算法程序的Java代码
时间: 2024-12-12 12:24:28 浏览: 17
RSA是一种非对称加密算法,它由Ron Rivest、Adi Shamir和Leonard Adleman于1977年发明。在Java中,RSA的实现通常涉及到密钥生成、加密、解密等操作。下面是一个简化的Java代码示例,展示了如何创建一个基本的RSA加密系统:
```java
import java.security.KeyPair;
import java.security.KeyPairGenerator;
import javax.crypto.Cipher;
public class RSAExample {
public static void main(String[] args) throws Exception {
// 生成RSA密钥对
KeyPairGenerator keyGen = KeyPairGenerator.getInstance("RSA");
keyGen.initialize(1024); // 选择密钥长度
KeyPair keyPair = keyGen.generateKeyPair();
// 获取公钥和私钥
Cipher cipher = Cipher.getInstance("RSA"); // 初始化加解密器
PublicKey publicKey = keyPair.getPublic();
PrivateKey privateKey = keyPair.getPrivate();
// 加密数据(使用公钥)
String originalMessage = "Hello, RSA!";
byte[] encryptedBytes = encrypt(cipher, publicKey, originalMessage.getBytes());
System.out.println("Encrypted message: " + new String(encryptedBytes));
// 解密数据(使用私钥)
byte[] decryptedBytes = decrypt(cipher, privateKey, encryptedBytes);
System.out.println("Decrypted message: " + new String(decryptedBytes));
}
// 加密函数
private static byte[] encrypt(Cipher cipher, PublicKey publicKey, byte[] data) throws Exception {
cipher.init(Cipher.ENCRYPT_MODE, publicKey);
return cipher.doFinal(data);
}
// 解密函数
private static byte[] decrypt(Cipher cipher, PrivateKey privateKey, byte[] encryptedData) throws Exception {
cipher.init(Cipher.DECRYPT_MODE, privateKey);
return cipher.doFinal(encryptedData);
}
}
```
这个例子中,我们首先生成一对RSA密钥,然后分别用于加密(使用公钥)和解密(使用私钥)。注意,实际应用中需要妥善处理文件I/O和异常处理。
阅读全文
相关推荐
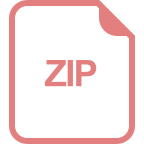
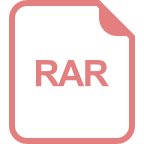
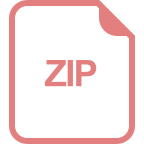
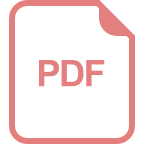
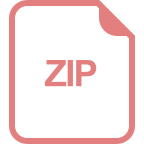
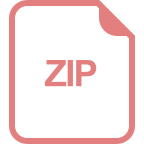
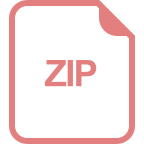
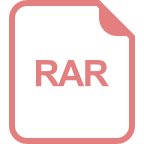
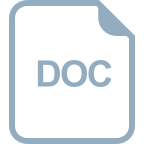
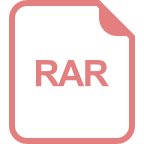
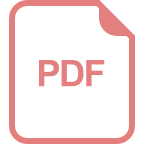
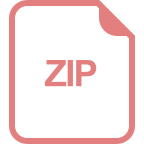
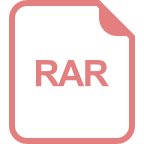
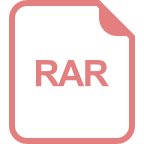
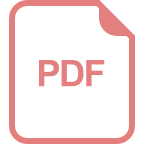
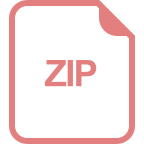
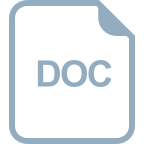
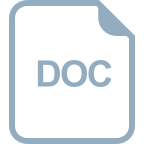
