c语言 pdf转图片
时间: 2024-01-13 10:18:31 浏览: 35
以下是使用ImageMagick将PDF转换为图片的C语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <wand/MagickWand.h>
int main(int argc, char **argv)
{
MagickWand *magick_wand;
MagickBooleanType status;
MagickPixelPacket pixel;
PixelIterator *iterator;
ExceptionInfo *exception;
char *pdf_file = "example.pdf";
char *image_file = "example.jpg";
int width, height;
MagickWandGenesis();
exception = AcquireExceptionInfo();
magick_wand = NewMagickWand();
status = MagickReadImage(magick_wand, pdf_file);
if (status == MagickFalse) {
CatchException(exception);
fprintf(stderr, "Failed to read PDF file %s\n", pdf_file);
return 1;
}
MagickResetIterator(magick_wand);
iterator = NewPixelIterator(magick_wand);
width = MagickGetImageWidth(magick_wand);
height = MagickGetImageHeight(magick_wand);
for (int y = 0; y < height; y++) {
PixelWand **pixels = PixelGetNextIteratorRow(iterator, &width);
for (int x = 0; x < width; x++) {
PixelGetMagickColor(pixels[x], &pixel);
// Do something with the pixel color
}
PixelSyncIterator(iterator);
}
status = MagickWriteImage(magick_wand, image_file);
if (status == MagickFalse) {
CatchException(exception);
fprintf(stderr, "Failed to write image file %s\n", image_file);
return 1;
}
magick_wand = DestroyMagickWand(magick_wand);
exception = DestroyExceptionInfo(exception);
MagickWandTerminus();
return 0;
}
```
上述代码使用ImageMagick的MagickWand API读取PDF文件并将其转换为JPEG格式的图像。你可以根据需要更改输出图像的格式。请注意,你需要安装ImageMagick库和开发文件才能编译此代码。
相关推荐
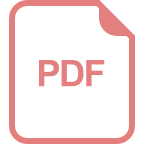














