vue2 通过滚轮对一张图片放大缩小,拖拽,图片可以恢复原来大小,代码怎么写
时间: 2024-10-22 07:09:38 浏览: 29
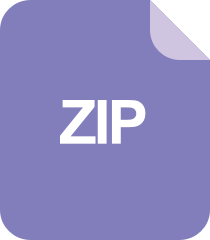
基于vue图片拖拽放大缩小查看npm包
在Vue2中,你可以使用`vue-touch`库来处理滚动、缩放和拖动事件,配合`v-model`绑定和计算属性,实现图片的动态操作。首先需要安装`vue-touch`:
```bash
npm install vue-touch
```
然后,在组件中引入并使用它:
```html
<template>
<div class="image-container">
<img :src="currentImage" :style="{ transform: 'scale(' + scale + ')' }" @wheel="handleWheel" @dragstart.prevent="handleDragStart" @dragend="handleDragEnd" />
</div>
</template>
<script>
import { useTouch } from 'vue-touch'
export default {
data() {
return {
currentImage: 'your-image-url',
scale: 1,
touch: null
}
},
mounted() {
this.touch = useTouch(this)
},
beforeDestroy() {
if (this.touch) {
this.touch.stop()
this.touch = null
}
},
methods: {
handleWheel(event) {
event.preventDefault()
const delta = event.deltaY
// 缩放图片
this.scale += delta / 100 // 可以调整这个比例,适应你的需求
},
handleDragStart(e) {
e.preventDefault()
this.startX = e.touches[0].clientX
this.startY = e.touches[0].clientY
},
handleDragEnd(e) {
e.preventDefault()
const endX = e.changedTouches[0].clientX
const endY = e.changedTouches[0].clientY
// 恢复到原始大小,假设中心点不变
const centerX = (this.startX + endX) / 2
const centerY = (this.startY + endY) / 2
const newScale = 1 // 这里设置回原始大小的缩放比例
this.scale = Math.min(Math.max(newScale, 1), this.maxZoom) // 如果有最大缩放限制,别忘了添加
this.startX = null
this.startY = null
}
},
computed: {
maxZoom: () => {
// 计算图片的最大缩放比例,如宽度比高度大,取宽度作为参考
return this.$el.clientWidth / this.currentImage.width
}
}
}
</script>
<style scoped>
.image-container {
position: relative;
overflow: hidden;
}
</style>
```
上面的代码实现了图片的滚动、缩放以及拖拽操作,并在结束时自动恢复原大小。记得替换`currentImage`变量为你实际的图片URL。
阅读全文
相关推荐
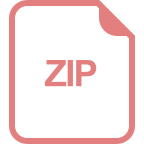
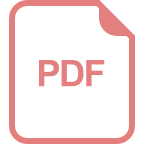




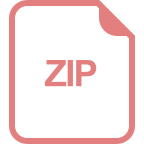
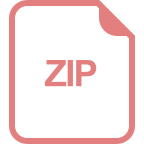
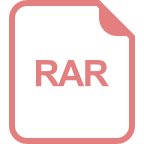
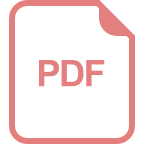
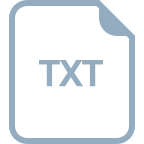
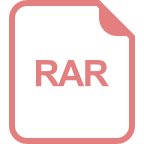


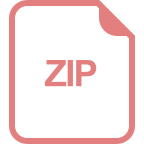
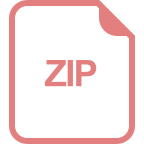
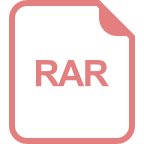
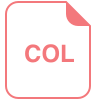
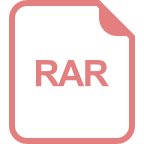