#ifndef _INC_STDLIB
时间: 2024-04-08 12:28:07 浏览: 17
#ifndef _INC_STDLIB是C/C++中的预处理指令,用于条件编译。它的作用是在编译过程中判断某个标识符是否已经被定义,如果没有被定义,则编译后面的代码块,否则跳过后面的代码块。
在C/C++中,头文件通常使用#ifndef _INC_XXX来避免重复包含。当一个头文件被多次包含时,为了避免重复定义变量、函数等问题,可以使用#ifndef _INC_XXX来判断该头文件是否已经被包含过。
具体使用方法如下:
```
#ifndef _INC_STDLIB
#define _INC_STDLIB
// 这里是需要包含的代码
#endif // _INC_STDLIB
```
这样,在第一次包含该头文件时,_INC_STDLIB还没有被定义,会执行#ifndef _INC_STDLIB后面的代码块,并定义_INC_STDLIB。当再次包含该头文件时,_INC_STDLIB已经被定义,会跳过#ifndef _INC_STDLIB后面的代码块。
相关问题
#ifndef _MAIN_C
#ifndef _MAIN_C 是一个条件编译指令,用于在编译过程中判断是否已经定义了 _MAIN_C 宏。如果没有定义,则编译下面的代码块,否则跳过。
这个指令通常用于防止头文件的重复包含,以避免编译错误。在一个程序中,如果多个源文件都包含了同一个头文件,就可能导致重复定义的问题。通过使用条件编译指令,可以确保头文件只被编译一次。
在 C 语言中,通常会在头文件的开头使用 #ifndef _HEADER_H 和 #define _HEADER_H 来定义一个宏,然后在文件的结尾使用 #endif 来结束条件编译块。
#ifndef __cplusplus
#ifndef __cplusplus是C++中的预处理指令,用于判断当前代码是否在C++环境下编译。如果在C++环境下编译,则执行#ifndef和#endif之间的代码;如果不在C++环境下编译,则忽略#ifndef和#endif之间的代码。
这个预处理指令通常用于在C++代码中包含C语言的头文件时进行判断,以确保C语言的头文件在C++环境下能够正确编译。
相关问题:
1. 为什么需要使用#ifndef __cplusplus?
2. C++和C语言有什么区别?
3. C++中的预处理指令还有哪些?
相关推荐
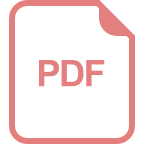












