在c#中,如何把 {"Param":{"Change_id":"befa641c0d2445c391f856ba55f419c0","Station_code_Physical":"P-PAL1-001","Ot":2}} 作为httpPost的请求参数提交
时间: 2024-06-16 18:01:36 浏览: 88
在C#中,当你想要将一个JSON对象如`{"Param":{"Change_id":"befa641c0d2445c391f856ba55f419c0","Station_code_Physical":"P-PAL1-001","Ot":2}}`作为HTTP POST请求的参数发送,你可以使用HttpClient或者第三方库(如Newtonsoft.Json或System.Text.Json)来序列化和发送数据。这里我将展示如何使用.NET框架中的HttpClient和System.Text.Json。
首先,确保已添加System.Text.Json NuGet包:
```bash
Install-Package System.Text.Json
```
然后,你可以按照以下步骤操作:
1. 创建一个类来映射JSON结构:
```csharp
public class RequestParams
{
public Dictionary<string, string> Param { get; set; }
}
```
2. 将JSON字符串转换为RequestParams对象:
```csharp
string jsonString = @"{""Param"":{""Change_id"":""befa641c0d2445c391f856ba55f419c0"",""Station_code_Physical"":""P-PAL1-001"",""Ot"":2}}";
RequestParams requestParams = JsonSerializer.Deserialize<RequestParams>(jsonString);
```
3. 使用HttpClient发送POST请求:
```csharp
using HttpClient httpClient = new HttpClient();
var content = new StringContent(JsonSerializer.Serialize(requestParams), Encoding.UTF8, "application/json");
Uri uri = new Uri("http://your-api-url.com/endpoint"); // 替换为你的实际API地址
HttpResponseMessage response = await httpClient.PostAsync(uri, content);
// 检查响应
response.EnsureSuccessStatusCode();
string responseBody = await response.Content.ReadAsStringAsync();
```
4. 发送请求后,`responseBody`将包含服务器返回的结果。
相关问题:
1. HttpClient是什么?
2. Newtonsoft.Json和System.Text.Json有何区别?
3. 如何处理HTTP响应的状态码和错误?
相关推荐
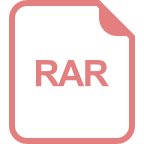

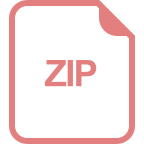
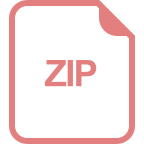
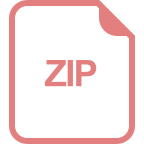
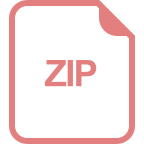
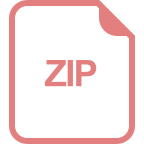
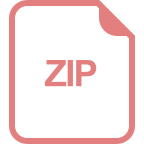
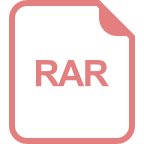
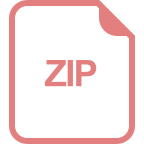




